Blazor Smart.Table Selection
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup the Blazor Smart.Table
Follow the Get Started with Smart.Table guide to set up the component.
Selection
This tutorial will show you how to use the Table's Selection functionality.
First, you need to set the Selection property of the Table to true
, like this:
<Table OnReady="OnTableReady" Selection>This will enable the default selection of the Table.
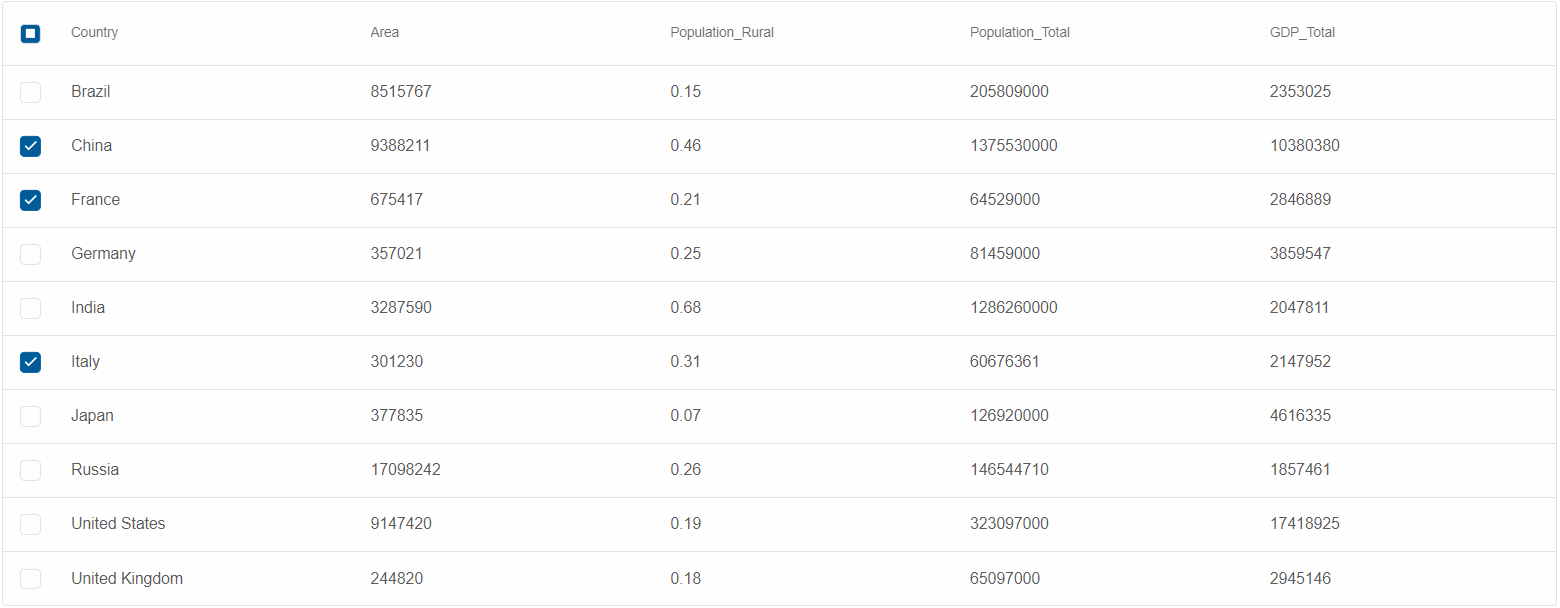
You can also set the SelectionMode property that is an enum with the following possible values:
TableSelectionMode.One
TableSelectionMode.Extended
TableSelectionMode.Many
Example
Here is a full example of Table with selection mode extended:
- Clicking on a checkbox selects a row and keeps the previous selection.
- Clicking anywhere on a row except on its checkbox deselects all other rows.
- Ctrl- and Shift-clicking selects multiple rows.
- Clicking a selected row or its checkbox deselects it.
<div> <Table OnReady="OnTableReady" Selection="true" SelectionMode="TableSelectionMode.Extended" KeyboardNavigation="true"> <table> <thead> <tr> <th scope="col">Country</th> <th scope="col">Area</th> <th scope="col">Population_Rural</th> <th scope="col">Population_Total</th> <th scope="col">GDP_Total</th> </tr> </thead> <tbody> <tr> <td>Brazil</td> <td>8515767</td> <td>0.15</td> <td>205809000</td> <td>2353025</td> </tr> <tr> <td>China</td> <td>9388211</td> <td>0.46</td> <td>1375530000</td> <td>10380380</td> </tr> <tr> <td>France</td> <td>675417</td> <td>0.21</td> <td>64529000</td> <td>2846889</td> </tr> <tr> <td>Germany</td> <td>357021</td> <td>0.25</td> <td>81459000</td> <td>3859547</td> </tr> <tr> <td>India</td> <td>3287590</td> <td>0.68</td> <td>1286260000</td> <td>2047811</td> </tr> <tr> <td>Italy</td> <td>301230</td> <td>0.31</td> <td>60676361</td> <td>2147952</td> </tr> <tr> <td>Japan</td> <td>377835</td> <td>0.07</td> <td>126920000</td> <td>4616335</td> </tr> <tr> <td>Russia</td> <td>17098242</td> <td>0.26</td> <td>146544710</td> <td>1857461</td> </tr> <tr> <td>United States</td> <td>9147420</td> <td>0.19</td> <td>323097000</td> <td>17418925</td> </tr> <tr> <td>United Kingdom</td> <td>244820</td> <td>0.18</td> <td>65097000</td> <td>2945146</td> </tr> </tbody> </table> </Table> </div> @code { private void OnTableReady(Table table) { table.Select(1); table.Select(2); table.Select(5); } }