Gantt Events
Smart.Gantt provides numerous events to help you develop custom behavior that fits your project's needs.
The events must first be attached as a property to the Grid, then specify the custom function they should execute.
Note that event.detail contains different information depening on the event
<h2> Gantt Chart </h2> <h3>Clicked Task Index: @clickedTask</h3> <GanttChart @ref="gantt" DataSource="@Records" OnItemClick="OnItemClick"/> @code { public partial class GanttDataRecord { [JsonPropertyName("label")] public string Label { get; set; } [JsonPropertyName("dateStart")] public string DateStart { get; set; } [JsonPropertyName("dateEnd")] public string DateEnd { get; set; } [JsonPropertyName("type")] public string Type { get; set; } [JsonPropertyName("duration")] public int Duration { get; set; } } GanttChart gantt; int clickedTask; private void OnItemClick(Event ev) { clickedTask = Int16.Parse(ev["Detail"].Id); } public List<GanttDataRecord> Records; protected override void OnInitialized() { Records = new List<GanttDataRecord>() { new GanttDataRecord{ Label = "Develop Website", DateStart = "2021-01-01", DateEnd = "2021-01-20", Type = "task" }, new GanttDataRecord{ Label = "Marketing Campaign", DateStart = "2021-01-05", DateEnd = "2021-01-15", Type = "task" }, new GanttDataRecord{ Label = "Publishing", DateStart = "2021-01-10", DateEnd = "2021-01-26", Type = "task" }, new GanttDataRecord{ Label = "Find clients", DateStart = "2021-01-12", DateEnd = "2021-01-25", Type = "task" } }; } }
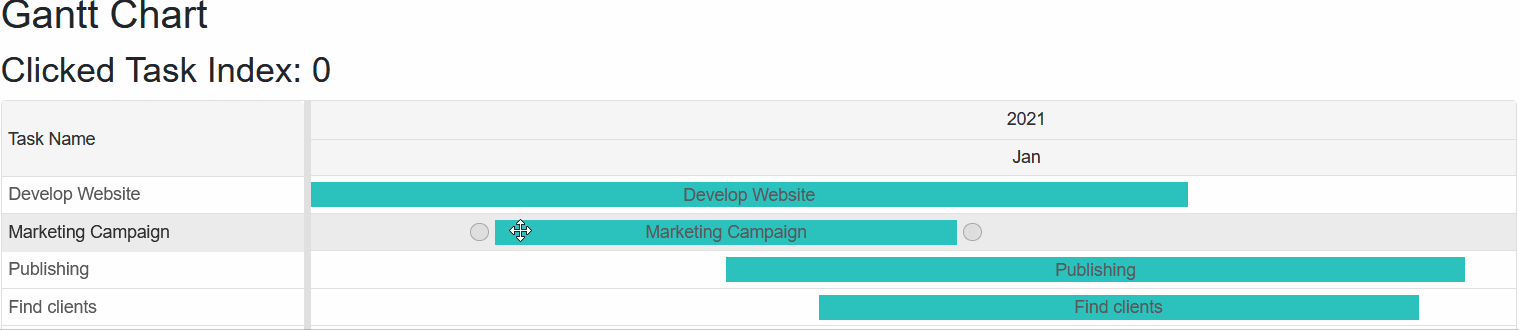
Timeline-related Events
OnConnectionStart
- triggered when the user starts connecting one task to another.
Event Detail
: dynamic startIndex
Note: This event allows to cancel the operation by calling event.preventDefault() in the event handler functionOnConnectionEnd
- triggered when the user completes a connection between two tasks.
Event Detail
: string id, dynamic startTaskId, dynamic startIndex, dynamic endIndex, dynamic endTaskId, dynamic typeOnChange
- triggered when a Task is selected/unselected.
Event Detail
: dynamic value, dynamic oldValueOnColumnResize
- triggered when a Tree column is resized.
Event Detail
: string dataField, dynamic headerCellElement, string widthInPercentages, string widthOnDragStart
- triggered when dragging of a task starts.
Event Detail
: string id, dynamic item, dynamic dateStart, dynamic dateEnd
Note: This event allows to cancel the operation by calling event.preventDefault() in the event handler functionOnDragEnd
- triggered when dragging of a task finishes.
Event Detail
: string id, dynamic item, dynamic dateStart, dynamic dateEndOnProgressChangeStart
- triggered when the progress of a task bar starts to change as a result of user interaction.
Event Detail
: string id, int index, dynamic progress
Note: This event allows to cancel the operation by calling event.preventDefault() in the event handler functionOnProgressChangeEnd
- triggered when the progress of a task is changed.
Event Detail
: string id, int index, dynamic progressOnResizeStart
- triggered when resizing of a task starts.
Event Detail
: string id, dynamic item, dynamic dateStart, dynamic dateEnd
Note: This event allows to cancel the operation by calling event.preventDefault() in the event handler functionOnResizeEnd
- triggered when the resizing of a task finishes.
Event Detail
: string id, dynamic item, dynamic dateStart, dynamic dateEnd
Editing Window-related Events
OnClosing
- triggered just before the window for task editing starts closing.
Event Detail
: dynamic target, dynamic type
Note: This event allows to cancel the operation by calling event.preventDefault() in the event handler functionOnClose
- triggered when the window for task editing is closed / hidden.
Event Detail
: N/AOnOpening
- triggered just before the window for task editing starts opening.
Event Detail
: dynamic target, dynamic type
Note: This event allows to cancel the operation by calling event.preventDefault() in the event handler functionOnOpen
- triggered when the window for task editing is open / visible.
Event Detail
: N/A
Items-related Events
OnCollapse
- triggered when an item is collapsed.
Event Detail
: dynamic isGroup, dynamic item, int index, string label, dynamic valueOnExpand
- triggered when an item is expanded.
Event Detail
: dynamic isGroup, dynamic item, int index, string label, dynamic valueOnItemClick
- triggered when a task, resource or connection is clicked inside the Timeline or the Tree columns.
Event Detail
: string id, dynamic item, dynamic type, dynamic originalEventOnItemInsert
- triggered when a Task/Resource/Connection is inserted.
Event Detail
: dynamic type, dynamic itemOnItemRemove
- triggered when a Task/Resource/Connection is removed.
Event Detail
: string id, dynamic type, dynamic itemOnItemUpdate
- triggered when a Task/Resource/Connection is updated.
Event Detail
: string id, dynamic type, dynamic item
General Events
OnBeginUpdate
- triggered when a batch update was started after executing thebeginUpdate
method.
Event Detail
: N/AOnEndUpdate
- triggered when a batch update was ended from after executing theendUpdate
method
Event Detail
: N/AOnFilter
- triggered when the GanttChart is filtered.
Event Detail
: dynamic type, dynamic action, dynamic filtersOnScrollBottomReached
- triggered when the Timeline has been scrolled to the bottom.
Event Detail
: N/AOnScrollTopReached
- triggered when the Timeline has been scrolled to the top.
Event Detail
: N/AOnScrollLeftReached
- triggered when the Timeline has been scrolled to the beginning (horizontally).
Event Detail
: N/AOnScrollRightReached
- triggered when the Timeline has been scrolled to the end (horizontally).
Event Detail
: N/A