Blazor - Get Started with Smart.MaskedTextBox
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic MaskedTextBox
Smart.MaskedTextBox is a custom input element that allows using a mask to distinguish between proper and improper user input.
The list of avaible options is set using the DataSource property.
- Add the MaskedTextBox component to the Pages/Index.razor file
<MaskedTextBox></MaskedTextBox>
- Create a new custom mask, using the # as a prompt character.
The prompt character can be modified with thePromptChar
property:<MaskedTextBox Label="Phone number" Mask="+1 (###) ### - ####"></MaskedTextBox>
- Default value can be set using the
Value
property:<MaskedTextBox Label="Phone number" Mask="+1 (###) ### - ####" Value="12314567890"></MaskedTextBox>

Copy & Paste
The behaviour when cutting or copying the value of the input can be customized with the CutCopyMaskFormat property:
<MaskedTextBox Label="Phone number" Mask="+1 (###) ### - ####" CutCopyMaskFormat="MaskedTextBoxCutCopyMaskFormat.IncludePromptAndLiterals"></MaskedTextBox>
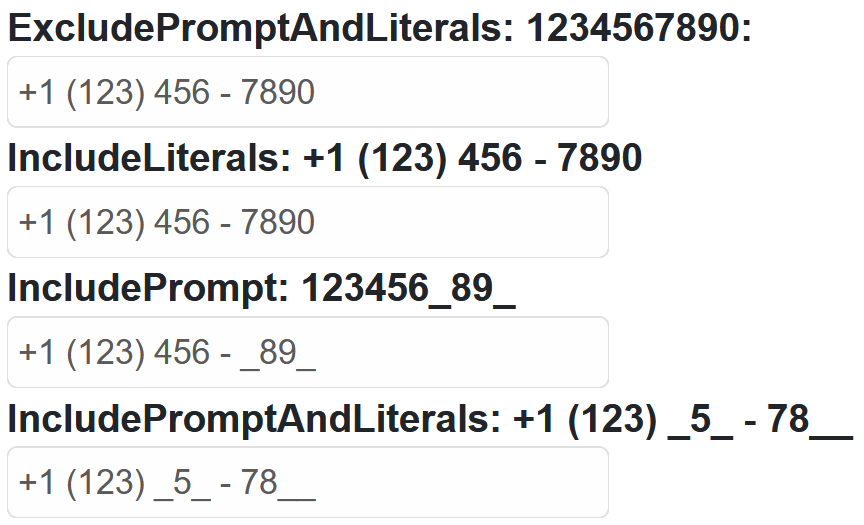
Overwrite Mode
The input can be set to Overwrite mode, which enables users to overwrite the input value, without deleting the value first.
<MaskedTextBox Label="Phone number" Mask="+1 (###) ### - ####" IsOverwriteMode="true"></MaskedTextBox>
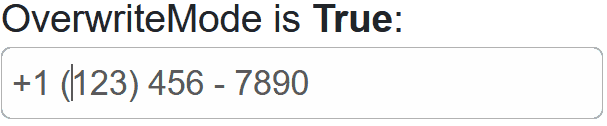
MaskInput Events
Smart.MaskInput provides multiple events that can help you expand the component's functionality.
Each event object has unique event.detail parameters.
OnChange
- triggered when the value of the Text Box is changed.
Event Details
: dynamic oldValue, dynamic valueOnChanging
- triggered on each key up event of the MaskedTextBox, if the value is changed.
Event Details
: dynamic oldValue, dynamic valueOnValidation
- triggered if the validation property is set. Indicates whether valiation has passed successfully or not.
Event Details
: dynamic success
The demo below uses the OnChange
Event to display the valu of the input field:
<h3 style="min-height:40px">@value</h3> <MaskedTextBox Label="Phone number" Mask="+1 (###) ### - ####" IsOverwriteMode="false" CutCopyMaskFormat="MaskedTextBoxCutCopyMaskFormat.IncludePromptAndLiterals" OnChanging="OnChanging"></MaskedTextBox> @code { public string value; private void OnChanging(Event ev){ if(ev.ContainsKey("Detail")){ MaskedTextBoxChangingEventDetail detail = ev["Detail"]; value = detail.Value; } } }
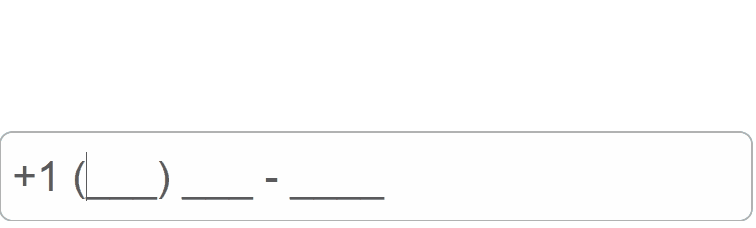
Two-way Value Binding
The MaskedTextBox component also supports two-way value binding. When a change in the value occurs, it is automatically reflected in the variable:
<h3>@textValue</h3> <Input @bind-Value = "@textValue"></Input> @code{ string textValue = ""; }
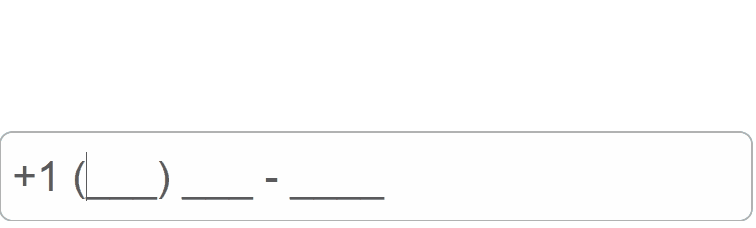