Blazor - Get Started with Smart.TimePicker
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Time Picker
Smart.TimePicker allows users to easily select Time values by using a circular clock for hours and minutes
- Add the TimePicker component to the Pages/Index.razor file
<TimePicker></TimePicker>
- Default value of the input is the current time. It can be changed using the Value property.
It accepts new Date() object or valid time in string format:
<TimePicker Value="@time"></TimePicker> @code{ string time = "09:20"; }
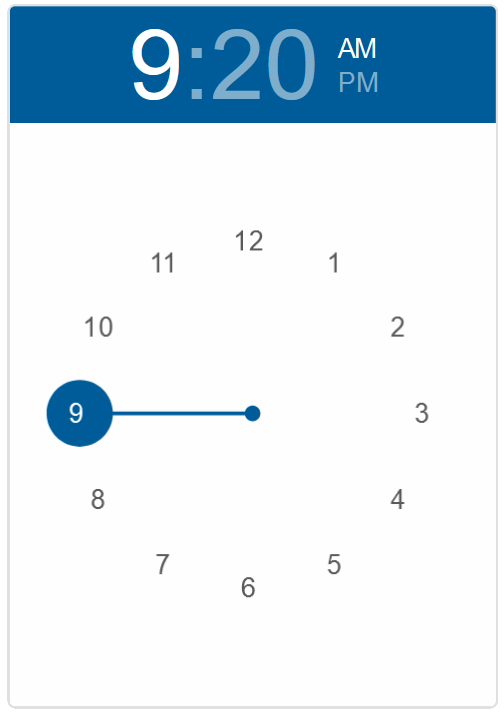
Landscape View
Depending on the screen orientation, Smart.TimePicker can be set to Landscape View.
<TimePicker Value="@time" View="ViewLayout.Landscape"></TimePicker>
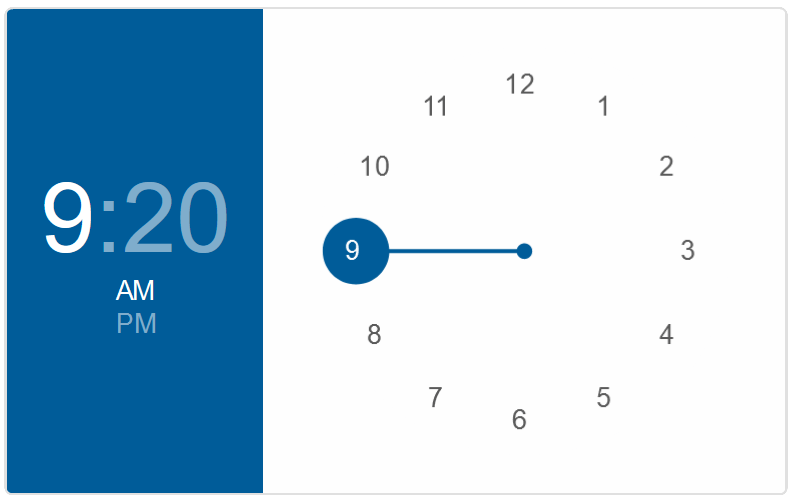
Time Format
By default, TimePicker displayes uses the 12H time format. This can be changed using the Format
property.
<TimePicker Value="@time" View="ViewLayout.Landscape" Format="TimePickerFormat.Two4Hour"></TimePicker>
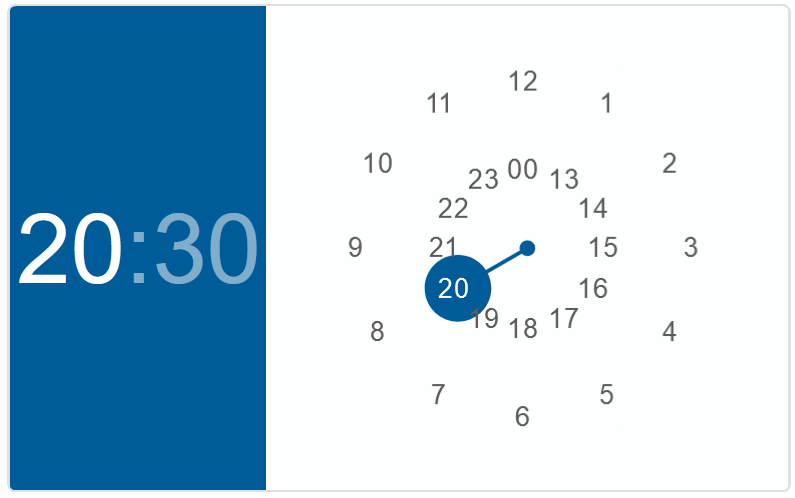
Minute Interval
The Minute Interval can be modfied to any integer value:
<TimePicker Value="@time" View="ViewLayout.Landscape" Format="TimePickerFormat.Two4Hour" MinuteInterval="10" AutoSwitchToMinutes></MultiComboInput>
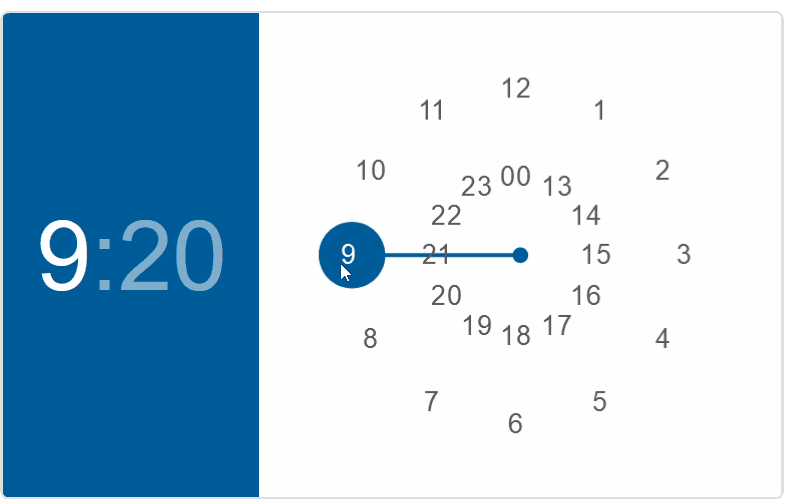
TimePicker Events
Smart.TimePicker provides an OnChange Event that can help you expand the component's
functionality.
The event object can have unique event.detail parameters.
OnChange
- triggered when the value is changed.
Event Details
: dynamic oldValue, dynamic value
The demo below uses the OnChange event to display the currently selected time in the UTC Timezone
<h3 style="min-height:40px">@selectedTime</h3> <TimePicker Value="@time" View="ViewLayout.Landscape" Format="TimePickerFormat.Two4Hour" AutoSwitchToMinutes OnChange="OnChange"></TimePicker> @code{ string time = "09:20"; string selectedTime; private void OnChange(Event ev){ TimePickerChangeEventDetail detail = ev["Detail"]; selectedTime = detail.Value.ToString("HH:mm") + " UTC"; } }
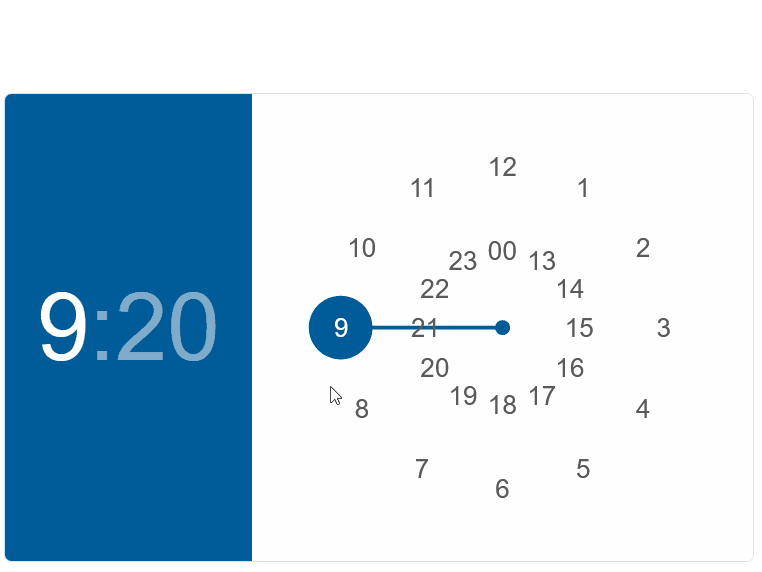