Build your web apps using Smart UI
Smart.CardView - configuration and usage
Overview
Smart.CardView represents a control for displaying tabular data. Each record of the data is represented by a data card which can contain multiple data fields and also show cover image(s) and a title.
Getting Started with CardView Web Component
Smart UI for Web Components is distributed as smart-webcomponents NPM package. You can also get the full download from our website with all demos from the Download page.Setup the CardView
Smart UI for Web Components is distributed as smart-webcomponents NPM package
- Download and install the package.
npm install smart-webcomponents
- Once installed, import the CardView module in your application.
<script type="module" src="node_modules/smart-webcomponents/source/modules/smart.cardview.js"></script>
-
Adding CSS reference
The smart.default.css CSS file should be referenced using following code.
<link rel="stylesheet" type="text/css" href="node_modules/smart-webcomponents/source/styles/smart.default.css" />
- Add the CardView tag to your Web Page
<smart-card-view id="cardview"></smart-card-view>
- Create the CardView Component
<script type="module"> Smart('#cardview', class { get properties() { return { dataSource: new Smart.DataAdapter({ dataSource: (function generateData(length) { const sampleData = [], firstNames = ['Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene'], lastNames = ['Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier'], petNames = ['Sam', 'Bob', 'Lucky', 'Tommy', 'Charlie', 'Olliver', 'Mixie', 'Fluffy', 'Acorn', 'Beak'], countries = ['Bulgaria', 'USA', 'UK', 'Singapore', 'Thailand', 'Russia', 'China', 'Belize'], productNames = ['Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Cramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist']; for (let i = 0; i < length; i++) { const row = {}; row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date(Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1)); row.petName = petNames[Math.floor(Math.random() * petNames.length)]; row.country = countries[Math.floor(Math.random() * countries.length)]; row.productName = productNames[Math.floor(Math.random() * productNames.length)]; row.price = parseFloat((Math.random() * (100 - 0.5) + 0.5).toFixed(2)); row.quantity = Math.round(Math.random() * (50 - 1) + 1); row.timeOfPurchase = new Date(2019, 0, 1, Math.round(Math.random() * 23), Math.round(Math.random() * (31 - 1) + 1)); row.expired = Math.random() >= 0.5; row.attachments = []; const maxAttachments = Math.floor(Math.random() * Math.floor(3)) + 1; for (let i = 0; i < maxAttachments; i++) { row.attachments.push("../../../images/travel/31.jpg"); } row.attachments = row.attachments.join(','); sampleData[i] = row; } return sampleData; }(50)), dataFields: [ 'firstName: string', 'lastName: string', 'birthday: date', 'petName: string', 'country: string', 'productName: string', 'price: number', 'quantity: number', 'timeOfPurchase: date', 'expired: boolean', 'attachments: string' ] }), columns: [ { label: 'First Name', dataField: 'firstName', icon: 'firstName' }, { label: 'Last Name', dataField: 'lastName', icon: 'lastName' }, { label: 'Birthday', dataField: 'birthday', icon: 'birthday', formatSettings: { formatString: 'd' } }, { label: 'Pet Name', dataField: 'petName', icon: 'petName' }, { label: 'Country', dataField: 'country', icon: 'country' }, { label: 'Product Name', dataField: 'productName', icon: 'productName' }, { label: 'Price', dataField: 'price', icon: 'price', formatSettings: { formatString: 'c2' } }, { label: 'Quantity', dataField: 'quantity', icon: 'quantity' }, { label: 'Time of Purchase', dataField: 'timeOfPurchase', icon: 'timeOfPurchase', formatSettings: { formatString: 'hh:mm tt' } }, { label: 'Expired', dataField: 'expired', icon: 'expired', formatFunction: function (settings) { settings.template = settings.value ? '☑' : '☐'; } }, { label: 'Attachments', dataField: 'attachments', image: true } ], coverField: 'attachments', titleField: 'firstName' } } }); </script>
Another option is to create the CardView is by using the traditional Javascript way:
const cardview = document.createElement('smart-card-view'); cardview.disabled = true; document.body.appendChild(cardview);
Smart framework provides a way to dynamically create a web component on demand from a DIV tag which is used as a host. The following imports the web component's module and creates it on demand, when the document is ready. The #cardview is the ID of a DIV tag.
import "../../source/modules/smart.cardview.js"; document.readyState === 'complete' ? init() : window.onload = init; function init() { const cardview = new Smart.CardView('#cardview', { dataSource: new Smart.DataAdapter({ dataSource: (function generateData(length) { const sampleData = [], firstNames = ['Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene'], lastNames = ['Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier'], petNames = ['Sam', 'Bob', 'Lucky', 'Tommy', 'Charlie', 'Olliver', 'Mixie', 'Fluffy', 'Acorn', 'Beak'], countries = ['Bulgaria', 'USA', 'UK', 'Singapore', 'Thailand', 'Russia', 'China', 'Belize'], productNames = ['Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Cramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist']; for (let i = 0; i < length; i++) { const row = {}; row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date(Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1)); row.petName = petNames[Math.floor(Math.random() * petNames.length)]; row.country = countries[Math.floor(Math.random() * countries.length)]; row.productName = productNames[Math.floor(Math.random() * productNames.length)]; row.price = parseFloat((Math.random() * (100 - 0.5) + 0.5).toFixed(2)); row.quantity = Math.round(Math.random() * (50 - 1) + 1); row.timeOfPurchase = new Date(2019, 0, 1, Math.round(Math.random() * 23), Math.round(Math.random() * (31 - 1) + 1)); row.expired = Math.random() >= 0.5; row.attachments = []; const maxAttachments = Math.floor(Math.random() * Math.floor(3)) + 1; for (let i = 0; i < maxAttachments; i++) { row.attachments.push("../../../images/travel/31.jpg"); } row.attachments = row.attachments.join(','); sampleData[i] = row; } return sampleData; }(50)), dataFields: [ 'firstName: string', 'lastName: string', 'birthday: date', 'petName: string', 'country: string', 'productName: string', 'price: number', 'quantity: number', 'timeOfPurchase: date', 'expired: boolean', 'attachments: string' ] }), columns: [ { label: 'First Name', dataField: 'firstName', icon: 'firstName' }, { label: 'Last Name', dataField: 'lastName', icon: 'lastName' }, { label: 'Birthday', dataField: 'birthday', icon: 'birthday', formatSettings: { formatString: 'd' } }, { label: 'Pet Name', dataField: 'petName', icon: 'petName' }, { label: 'Country', dataField: 'country', icon: 'country' }, { label: 'Product Name', dataField: 'productName', icon: 'productName' }, { label: 'Price', dataField: 'price', icon: 'price', formatSettings: { formatString: 'c2' } }, { label: 'Quantity', dataField: 'quantity', icon: 'quantity' }, { label: 'Time of Purchase', dataField: 'timeOfPurchase', icon: 'timeOfPurchase', formatSettings: { formatString: 'hh:mm tt' } }, { label: 'Expired', dataField: 'expired', icon: 'expired', formatFunction: function (settings) { settings.template = settings.value ? '☑' : '☐'; } }, { label: 'Attachments', dataField: 'attachments', image: true } ], coverField: 'attachments', titleField: 'firstName' }); }
- Open the page in your web server.
Cover and Title Fields
Smart.CardView displays the data passed to the dataSource property from the data fields present in the columns property/array. Some data fields can be highlighted in the form of card cover and title by setting the properties coverField and titleField respectively.
To be able to set a data field as a coverField, its respective column entry must have image set to true. The expected value of an "image" data field is a string that represent a list of image URLs, e.g.: "../../images/travel/34.jpg, ../../images/travel/17.jpg, ../../images/travel/1.jpg". The size of images in the cards' cover carousel can be set via the property coverMode to 'crop' or 'fit'.
<!DOCTYPE html> <head> <link rel="stylesheet" type="text/css" href=../../source/styles/smart.default.css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.button.js"></script> <script type="text/javascript" src="../../source/smart.card.js"></script> <script type="text/javascript" src="../../source/smart.carousel.js"></script> <script type="text/javascript" src="../../source/smart.checkbox.js"></script> <script type="text/javascript" src="../../source/smart.sortable.js"></script> <script type="text/javascript" src="../../source/smart.date.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.dropdownlist.js"></script> <script type="text/javascript" src="../../source/smart.listbox.js"></script> <script type="text/javascript" src="../../source/smart.tooltip.js"></script> <script type="text/javascript" src="../../source/smart.calendar.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> <script type="text/javascript" src="../../source/smart.datetimepicker.js"></script> <script type="text/javascript" src="../../source/smart.filter.js"></script> <script type="text/javascript" src="../../source/smart.input.js"></script> <script type="text/javascript" src="../../source/smart.complex.js"></script> <script type="text/javascript" src="../../source/smart.numerictextbox.js"></script> <script type="text/javascript" src="../../source/smart.gridpanel.js"></script> <script type="text/javascript" src="../../source/smart.data.js"></script> <script type="text/javascript" src="../../source/smart.scrollbar.js"></script> <script type="text/javascript" src="../../source/smart.switchbutton.js"></script> <script type="text/javascript" src="../../source/smart.window.js"></script> <script type="text/javascript" src="../../source/smart.cardview.js"></script> <script type="text/javascript" src="../../source/smart.tickintervalhandler.js"></script> <script type="text/javascript" src="../../source/smart.tank.js"></script> <script type="text/javascript" src="../../source/smart.slider.js"></script> <script type="text/javascript"> function generateData(length) { const sampleData = [], firstNames = ['Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene'], lastNames = ['Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier'], countries = ['Bulgaria', 'USA', 'UK', 'Singapore', 'Thailand', 'Russia', 'China', 'Belize']; for (let i = 0; i < length; i++) { const row = {}; row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date(Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1)); row.country = countries[Math.floor(Math.random() * countries.length)]; row.attachments = [`../../images/travel/${Math.floor(Math.random() * 36) + 1}.jpg, ../../ images / travel / ${Math.floor(Math.random() * 36) + 1}.jpg`]; sampleData[i] = row; } return sampleData; } Smart('#cardView', class { get properties() { return { dataSource: new Smart.DataAdapter( { dataSource: generateData(50), dataFields: [ 'firstName: string', 'lastName: string', 'birthday: date', 'country: string', 'attachments: string' ] }), columns: [ { label: 'First Name', dataField: 'firstName', icon: 'firstName' }, { label: 'Last Name', dataField: 'lastName', icon: 'lastName' }, { label: 'Birthday', dataField: 'birthday', icon: 'birthday', formatSettings: { formatString: 'd' } }, { label: 'Country', dataField: 'country', icon: 'country' }, { label: 'Attachments', dataField: 'attachments', image: true } ], coverField: 'attachments', titleField: 'firstName' }; } }); </script> </head> <body> <smart-card-view id="cardView"></smart-card-view> </body> </html>
Demo
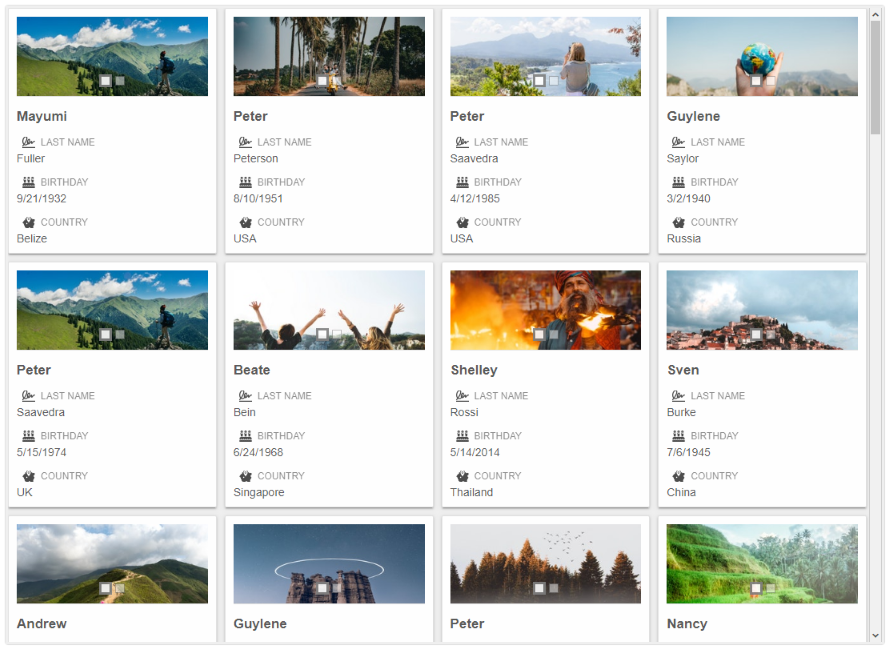
Resizing
Smart.CardView is built upon the CSS Grid Layout technology which allows the component to seemlessly resize and recalculate the necessary number of cards per row. This makes CardView particularly useful when viewed on mobile devices.
Editing
When the property editable is set to true, card data can be modified in an edit dialog. Editor elements are dynamically created based on dataSource and columns settings. The edit dialog also allows directly navigating to other cards by pressing the "Previous record" and "Next record" buttons in its header.
Demo
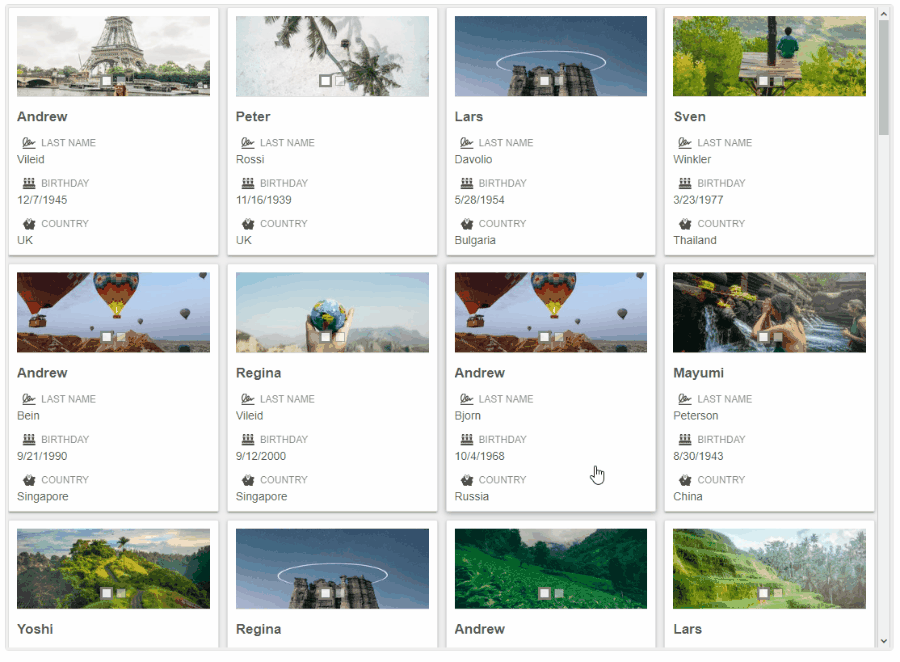
Customization, Filtering, Sorting, and Searching
When the CardView header is enabled (headerPosition is set to 'top' or 'bottom'), four buttons for card customization become available:
-
"Customize cards" - allows changing the cover field, the cover crop mode, reordering columns, and toggling their visibility.
-
"Filter" - allows filtering the CardView data by multiple data fields and criteria.
-
"Sort" - allows sorting the CardView data by multiple data fields.
-
Search icon - allows searching and highlighting items that contain specified keywords.
Collapsible Cards
By enabling the collapsible option, cards can be collapsed and expanded by clicking the arrow next to the card's title.
Scrolling
Smart.CardView offers four scrolling modes (configurable through the property scrolling):
- 'physical' - default scrolling.
- 'deferred' - when scrolling, view is not updated until the scrollbar's thumb is released.
- 'virtual' - server-bound scrolling. Loads records on demand when the element is scrolled to their position.
- 'infinite' - server-bound scrolling. Loads only some records initially. Additional records are dynamically loaded when the scrollbar reaches the bottom of the element.
Dragging Cards
When the property allowDrag is set to true, cards can be reordered by dragging and dropping.
Demo
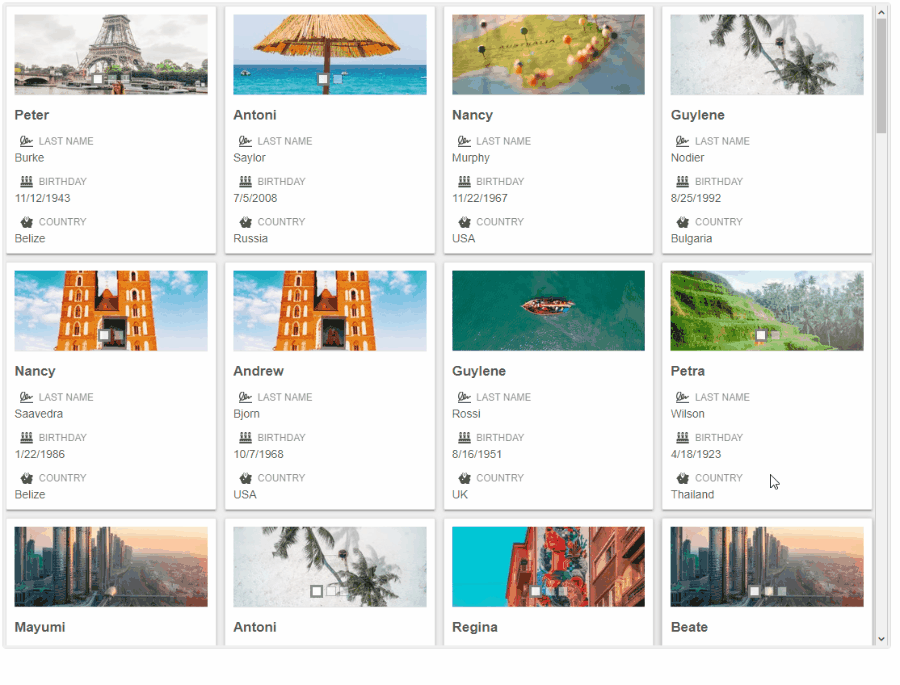
Methods
Smart.CardView has the following methods:
- addFilter(filters, operator) - adds filtering.
- addRecord(recordId, data,position) - adds a new record.
- addSort(dataFields, orderBy) - adds sorting.
- beginEdit(recordId) - begins an edit operation.
- cancelEdit() - ends the current edit operation and discards changes.
- closePanel() - closes any open header panel (drop down).
- endEdit() - ends the current edit operation and saves changes.
- ensureVisible(recordId) - makes sure a record is visible by scrolling to it.
- hideColumn(dataField) - hides a column.
- openCustomizePanel() - opens the "Customize cards" header panel (drop down).
- openFilterPanel() - opens the "Filter" header panel (drop down).
- openSortPanel() - opens the "Sort" header panel (drop down).
- removeFilter() - removes filtering.
- removeRecord(recordId) - removes a record.
- removeSort() - removes sorting.
- showColumn(dataField) - shows a column.
Create, Append, Remove, Get/Set Property, Invoke Method, Bind to Event
Create a new element:
const cardview = document.createElement('smart-card-view');
Append it to the DOM:
document.body.appendChild(cardview);
Remove it from the DOM:
cardview.parentNode.removeChild(cardview);
Set a property:
cardview.propertyName = propertyValue;
Get a property value:
const propertyValue = cardview.propertyName;
Invoke a method:
cardview.methodName(argument1, argument2);
Add Event Listener:
const eventHandler = (event) => { // your code here. }; cardview.addEventListener(eventName, eventHandler);
Remove Event Listener:
cardview.removeEventListener(eventName, eventHandler, true);
Using with Typescript
Smart Web Components package includes TypeScript definitions which enables strongly-typed access to the Smart UI Components and their configuration.
Inside the download package, the typescript directory contains .d.ts file for each web component and a smart.elements.d.ts typescript definitions file for all web components. Copy the typescript definitions file to your project and in your TypeScript file add a reference to smart.elements.d.ts
Read more about using Smart UI with Typescript.Getting Started with Angular CardView Component
Setup Angular Environment
Angular provides the easiest way to set angular CLI projects using Angular CLI tool.
Install the CLI application globally to your machine.
npm install -g @angular/cli
Create a new Application
ng new smart-angular-cardview
Navigate to the created project folder
cd smart-angular-cardview
Setup the CardView
Smart UI for Angular is distributed as smart-webcomponents-angular NPM package
- Download and install the package.
npm install smart-webcomponents-angular
- Once installed, import the CardViewModule in your application root or feature module.
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { CardViewModule } from 'smart-webcomponents-angular/cardview'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, CardViewModule ], bootstrap: [ AppComponent ] }) export class AppModule { }
- Adding CSS reference
The following CSS file is available in ../node_modules/smart-webcomponents-angular/ package folder. This can be referenced in [src/styles.css] using following code.@import 'smart-webcomponents-angular/source/styles/smart.default.css';
Another way to achieve the same is to edit the angular.json file and in the styles add the style."styles": [ "node_modules/smart-webcomponents-angular/source/styles/smart.default.css" ]
-
Example
app.component.html
<div class="demo-description">In Card View, data source records are represented as cards. Each Card contain content and actions about a single subject. smartCardView supports data sort, data filtering, data editing, data grouping and data searching.</div> <smart-card-view [columns]="columns" [titleField]="titleField" [dataSource]="dataSource"[coverField]="coverField" #cardview id="cardView"></smart-card-view>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { CardViewComponent, Smart } from 'smart-webcomponents-angular/cardview'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('cardview', { read: CardViewComponent, static: false }) cardview!: CardViewComponent; generateData(length: number): any[] { const sampleData = [], firstNames = ['Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene'], lastNames = ['Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier'], petNames = ['Sam', 'Bob', 'Lucky', 'Tommy', 'Charlie', 'Olliver', 'Mixie', 'Fluffy', 'Acorn', 'Beak'], countries = ['Bulgaria', 'USA', 'UK', 'Singapore', 'Thailand', 'Russia', 'China', 'Belize'], productNames = ['Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Cramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist']; for (let i = 0; i < length; i++) { const row: any = {}; row.firstName = (i + 1) + '. ' + firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date(Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1)); row.petName = petNames[Math.floor(Math.random() * petNames.length)]; row.country = countries[Math.floor(Math.random() * countries.length)]; row.productName = productNames[Math.floor(Math.random() * productNames.length)]; row.price = parseFloat((Math.random() * (100 - 0.5) + 0.5).toFixed(2)); row.quantity = Math.round(Math.random() * (50 - 1) + 1); row.timeOfPurchase = new Date(2019, 0, 1, Math.round(Math.random() * 23), Math.round(Math.random() * (31 - 1) + 1)); row.expired = Math.random() >= 0.5; row.attachments = []; const maxAttachments = Math.floor(Math.random() * Math.floor(3)) + 1; for (let i = 0; i < maxAttachments; i++) { row.attachments.push(`../../../images/travel/${Math.floor(Math.random() * 36) + 1}.jpg`); } row.attachments = row.attachments.join(','); sampleData[i] = row; } return sampleData; } dataSource = new Smart.DataAdapter({ dataSource: this.generateData(50), dataFields: [ 'firstName: string', 'lastName: string', 'birthday: date', 'petName: string', 'country: string', 'productName: string', 'price: number', 'quantity: number', 'timeOfPurchase: date', 'expired: boolean', 'attachments: string' ] }); columns = [ { label: 'First Name', dataField: 'firstName', icon: 'firstName' }, { label: 'Last Name', dataField: 'lastName', icon: 'lastName' }, { label: 'Birthday', dataField: 'birthday', icon: 'birthday', formatSettings: { formatString: 'd' } }, { label: 'Pet Name', dataField: 'petName', icon: 'petName' }, { label: 'Country', dataField: 'country', icon: 'country' }, { label: 'Product Name', dataField: 'productName', icon: 'productName' }, { label: 'Price', dataField: 'price', icon: 'price', formatSettings: { formatString: 'c2' } }, { label: 'Quantity', dataField: 'quantity', icon: 'quantity', formatFunction: function (settings) { const value = settings.value; let className; if (value < 20) { className = 'red'; } else if (value < 35) { className = 'yellow'; } else { className = 'green'; } settings.template = `<div class="${className}">${value}</div>`; } }, { label: 'Time of Purchase', dataField: 'timeOfPurchase', icon: 'timeOfPurchase', formatSettings: { formatString: 'hh:mm tt' } }, { label: 'Expired', dataField: 'expired', icon: 'expired', formatFunction: function (settings) { settings.template = settings.value ? '☑' : '☐'; } }, { label: 'Attachments', dataField: 'attachments', image: true } ]; coverField: string = 'attachments'; titleField: string = 'firstName' ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code. } }
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { CardViewModule } from 'smart-webcomponents-angular/cardview'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, CardViewModule ], bootstrap: [ AppComponent ] }) export class AppModule { }
Running the Angular application
After completing the steps required to render a CardView, run the following command to display the output in your web browser
ng serveand open localhost:4200 in your favorite web browser.
Read more about using Smart UI for Angular: https://www.htmlelements.com/docs/angular-cli/.
Getting Started with React CardView Component
Setup React Environment
The easiest way to start with React is to use create-react-app. To scaffold your project structure, follow the installation instructions.
npx create-react-app my-app cd my-app npm start
Preparation
Open src/App.js andsrc/App.css
- Remove everything inside the App div tag in src/App.js:
<div className="App"> </div>
- Remove the logo.svg import
- Remove the contents of src/App.css
- Remove src/logo.svg
Setup the CardView
Smart UI for React is distributed as smart-webcomponents-react NPM package
- Download and install the package.
npm install smart-webcomponents-react
Once installed, import the React CardView Component and CSS files in your application and render it
app.js
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React from "react"; import ReactDOM from 'react-dom/client'; import { Smart, CardView } from 'smart-webcomponents-react/cardview'; const App = () => { const generateData = (length) => { const sampleData = [], firstNames = ['Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene'], lastNames = ['Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier'], petNames = ['Sam', 'Bob', 'Lucky', 'Tommy', 'Charlie', 'Olliver', 'Mixie', 'Fluffy', 'Acorn', 'Beak'], countries = ['Bulgaria', 'USA', 'UK', 'Singapore', 'Thailand', 'Russia', 'China', 'Belize'], productNames = ['Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Cramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist']; for (let i = 0; i < length; i++) { const row = {}; row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date(Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1)); row.petName = petNames[Math.floor(Math.random() * petNames.length)]; row.country = countries[Math.floor(Math.random() * countries.length)]; row.productName = productNames[Math.floor(Math.random() * productNames.length)]; row.price = parseFloat((Math.random() * (100 - 0.5) + 0.5).toFixed(2)); row.quantity = Math.round(Math.random() * (50 - 1) + 1); row.timeOfPurchase = new Date(2019, 0, 1, Math.round(Math.random() * 23), Math.round(Math.random() * (31 - 1) + 1)); row.expired = Math.random() >= 0.5; row.attachments = []; const maxAttachments = Math.floor(Math.random() * Math.floor(3)) + 1; for (let i = 0; i < maxAttachments; i++) { row.attachments.push(`https://www.htmlelements.com/demos//images/travel/${Math.floor(Math.random() * 36) + 1}.jpg`); } row.attachments = row.attachments.join(','); sampleData[i] = row; } return sampleData; } const columns = [{ label: 'First Name', dataField: 'firstName', icon: 'firstName' }, { label: 'Last Name', dataField: 'lastName', icon: 'lastName' }, { label: 'Birthday', dataField: 'birthday', icon: 'birthday', formatSettings: { formatString: 'd' } }, { label: 'Pet Name', dataField: 'petName', icon: 'petName' }, { label: 'Country', dataField: 'country', icon: 'country' }, { label: 'Product Name', dataField: 'productName', icon: 'productName' }, { label: 'Price', dataField: 'price', icon: 'price', formatSettings: { formatString: 'c2' } }, { label: 'Quantity', dataField: 'quantity', icon: 'quantity', formatFunction: function (settings) { const value = settings.value; let className; if (value < 20) { className = 'red'; } else if (value < 35) { className = 'yellow'; } else { className = 'green'; } settings.template = `<div className="${className}">${value}</div>`; } }, { label: 'Time of Purchase', dataField: 'timeOfPurchase', icon: 'timeOfPurchase', formatSettings: { formatString: 'hh:mm tt' } }, { label: 'Expired', dataField: 'expired', icon: 'expired', formatFunction: function (settings) { settings.template = settings.value ? '☑' : '☐'; } }, { label: 'Attachments', dataField: 'attachments', image: true } ]; const dataSource = generateData(50); const dataSourceSettings = { dataFields: [ 'firstName: string', 'lastName: string', 'birthday: date', 'petName: string', 'country: string', 'productName: string', 'price: number', 'quantity: number', 'timeOfPurchase: date', 'expired: boolean', 'attachments: string' ] }; return ( <div> <div className="demo-description">In Card View, data source records are represented as cards. Each Card contain content and actions about a single subject. smartCardView supports data sort, data filtering, data editing, data grouping and data searching.</div> <CardView coverField="attachments" titleField="firstName" id="cardView" dataSourceSettings={dataSourceSettings} dataSource={dataSource} columns={columns}> </CardView> </div> ); } export default App;
Running the React application
Start the app withnpm startand open localhost:3000 in your favorite web browser to see the output.
Read more about using Smart UI for React: https://www.htmlelements.com/docs/react/.
Getting Started with Vue CardView Component
Setup Vue Environment
We will use vue-cli to get started. Let's install vue-cli
npm install -g @vue/cli
Then we can start creating our Vue.js projects with:
vue create my-project
Setup the CardView
Open the "my-project" folder and run:
npm install smart-webcomponents
Setup with Vue 3.x
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open vite.config.js in your favorite text editor and change its contents to the following:
vite.config.js
import { fileURLToPath, URL } from 'node:url' import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' // https://vitejs.dev/config/ export default defineConfig({ plugins: [ vue({ template: { compilerOptions: { isCustomElement: tag => tag.startsWith('smart-') } } }) ], resolve: { alias: { '@': fileURLToPath(new URL('./src', import.meta.url)) } } })
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <div class="vue-root"> <div class="demo-description"> In Card View, data source records are represented as cards. Each Card contain content and actions about a single subject. smartCardView supports data sort, data filtering, data editing, data grouping and data searching. </div> <smart-card-view id="cardView"></smart-card-view> </div> </template> <script> import { onMounted } from "vue"; import "smart-webcomponents/source/styles/smart.default.css"; import "smart-webcomponents/source/modules/smart.card.js"; import "smart-webcomponents/source/modules/smart.cardview.js"; export default { name: "app", setup() { onMounted(() => { function generateData(length) { const sampleData = [], firstNames = [ "Andrew", "Nancy", "Shelley", "Regina", "Yoshi", "Antoni", "Mayumi", "Ian", "Peter", "Lars", "Petra", "Martin", "Sven", "Elio", "Beate", "Cheryl", "Michael", "Guylene" ], lastNames = [ "Fuller", "Davolio", "Burke", "Murphy", "Nagase", "Saavedra", "Ohno", "Devling", "Wilson", "Peterson", "Winkler", "Bein", "Petersen", "Rossi", "Vileid", "Saylor", "Bjorn", "Nodier" ], petNames = [ "Sam", "Bob", "Lucky", "Tommy", "Charlie", "Olliver", "Mixie", "Fluffy", "Acorn", "Beak" ], countries = [ "Bulgaria", "USA", "UK", "Singapore", "Thailand", "Russia", "China", "Belize" ], productNames = [ "Black Tea", "Green Tea", "Caffe Espresso", "Doubleshot Espresso", "Caffe Latte", "White Chocolate Mocha", "Cramel Latte", "Caffe Americano", "Cappuccino", "Espresso Truffle", "Espresso con Panna", "Peppermint Mocha Twist" ]; for (let i = 0; i < length; i++) { const row = {}; row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date( Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1) ); row.petName = petNames[Math.floor(Math.random() * petNames.length)]; row.country = countries[Math.floor(Math.random() * countries.length)]; row.productName = productNames[Math.floor(Math.random() * productNames.length)]; row.price = parseFloat( (Math.random() * (100 - 0.5) + 0.5).toFixed(2) ); row.quantity = Math.round(Math.random() * (50 - 1) + 1); row.timeOfPurchase = new Date( 2019, 0, 1, Math.round(Math.random() * 23), Math.round(Math.random() * (31 - 1) + 1) ); row.expired = Math.random() >= 0.5; row.attachments = []; const maxAttachments = Math.floor(Math.random() * Math.floor(3)) + 1; for (let i = 0; i < maxAttachments; i++) { row.attachments.push( `../../../images/travel/${Math.floor(Math.random() * 36) + 1}.jpg` ); } row.attachments = row.attachments.join(","); sampleData[i] = row; } return sampleData; } window.Smart( "#cardView", class { get properties() { return { dataSource: new window.Smart.DataAdapter({ dataSource: generateData(50), dataFields: [ "firstName: string", "lastName: string", "birthday: date", "petName: string", "country: string", "productName: string", "price: number", "quantity: number", "timeOfPurchase: date", "expired: boolean", "attachments: string" ] }), columns: [ { label: "First Name", dataField: "firstName", icon: "firstName" }, { label: "Last Name", dataField: "lastName", icon: "lastName" }, { label: "Birthday", dataField: "birthday", icon: "birthday", formatSettings: { formatString: "d" } }, { label: "Pet Name", dataField: "petName", icon: "petName" }, { label: "Country", dataField: "country", icon: "country" }, { label: "Product Name", dataField: "productName", icon: "productName" }, { label: "Price", dataField: "price", icon: "price", formatSettings: { formatString: "c2" } }, { label: "Quantity", dataField: "quantity", icon: "quantity", formatFunction: function(settings) { const value = settings.value; let className; if (value < 20) { className = "red"; } else if (value < 35) { className = "yellow"; } else { className = "green"; } settings.template = `<div class="${className}">${value}</div>`; } }, { label: "Time of Purchase", dataField: "timeOfPurchase", icon: "timeOfPurchase", formatSettings: { formatString: "hh:mm tt" } }, { label: "Expired", dataField: "expired", icon: "expired", formatFunction: function(settings) { settings.template = settings.value ? "☑" : "☐"; } }, { label: "Attachments", dataField: "attachments", image: true } ], coverField: "attachments", titleField: "firstName" }; } } ); }); } }; </script> <style> .firstName:after { background-image: url("https://img.icons8.com/ios/16/000000/user-filled.png"); } .lastName:after { background-image: url("https://img.icons8.com/ios/16/000000/signature-filled.png"); } .birthday:after { background-image: url("https://img.icons8.com/ios/16/000000/birthday-filled.png"); } .petName:after { background-image: url("https://img.icons8.com/ios/16/000000/pet-commands-follow-filled.png"); } .productName:after { background-image: url("https://img.icons8.com/ios/16/000000/box-filled.png"); } .price:after { background-image: url("https://img.icons8.com/ios/16/000000/price-tag-euro-filled.png"); } .quantity:after { background-image: url("https://img.icons8.com/ios/16/000000/negative-dynamic-filled.png"); } .country:after { background-image: url("https://img.icons8.com/ios/16/000000/country-filled.png"); } .timeOfPurchase:after { background-image: url("https://img.icons8.com/ios/16/000000/alarm-clock-filled.png"); } .expired:after { background-image: url("https://img.icons8.com/ios/16/000000/expired-filled.png"); } .red, .yellow, .green { display: inline-block; border-radius: 10px; padding: 5px; } .red { background-color: #e94f37; color: white; } .yellow { background-color: #ffee93; } .green { background-color: #63c7b2; color: white; } </style>
We can now use the smart-card-view with Vue 3. Data binding and event handlers will just work right out of the box.
Setup with Vue 2.x
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open src/main.js in your favorite text editor and change its contents to the following:
main.js
import Vue from 'vue' import App from './App.vue' Vue.config.productionTip = false Vue.config.ignoredElements = [ 'smart-card-view' ] new Vue({ render: h => h(App), }).$mount('#app')
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <smart-card-view id="cardView"></smart-card-view> </template> <script> // import 'smart-webcomponents/source/modules/smart.grid.js'; // import 'smart-webcomponents/source/modules/smart.button.js'; // import 'smart-webcomponents/source/modules/smart.carousel.js'; // import 'smart-webcomponents/source/modules/smart.card.js'; // import 'smart-webcomponents/source/smart.gridpanel.js'; import 'smart-webcomponents/source/modules/smart.cardview.js'; // import "smart-webcomponents/source/smart.elements.js"; import "smart-webcomponents/source/styles/smart.default.css"; export default { name: "app", mounted: function() { function generateData(length) { const sampleData = [], firstNames = ['Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene'], lastNames = ['Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier'], petNames = ['Sam', 'Bob', 'Lucky', 'Tommy', 'Charlie', 'Olliver', 'Mixie', 'Fluffy', 'Acorn', 'Beak'], countries = ['Bulgaria', 'USA', 'UK', 'Singapore', 'Thailand', 'Russia', 'China', 'Belize'], productNames = ['Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Cramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist']; for (let i = 0; i < length; i++) { const row = {}; row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.birthday = new Date(Math.round(Math.random() * (2018 - 1918) + 1918), Math.round(Math.random() * 11), Math.round(Math.random() * (31 - 1) + 1)); row.petName = petNames[Math.floor(Math.random() * petNames.length)]; row.country = countries[Math.floor(Math.random() * countries.length)]; row.productName = productNames[Math.floor(Math.random() * productNames.length)]; row.price = parseFloat((Math.random() * (100 - 0.5) + 0.5).toFixed(2)); row.quantity = Math.round(Math.random() * (50 - 1) + 1); row.timeOfPurchase = new Date(2019, 0, 1, Math.round(Math.random() * 23), Math.round(Math.random() * (31 - 1) + 1)); row.expired = Math.random() >= 0.5; row.attachments = []; const maxAttachments = Math.floor(Math.random() * Math.floor(3)) + 1; for (let i = 0; i < maxAttachments; i++) { row.attachments.push(`https://loremflickr.com/320/240/nature?lock=${Math.floor(Math.random() * Math.floor(1000)) + 1}`); } row.attachments = row.attachments.join(','); sampleData[i] = row; } return sampleData; }; Smart( "#cardView", class { get properties() { return { dataSource: new Smart.DataAdapter({ dataSource: generateData(50), dataFields: [ "firstName: string", "lastName: string", "birthday: date", "petName: string", "country: string", "productName: string", "price: number", "quantity: number", "timeOfPurchase: date", "expired: boolean", "attachments: string" ] }), columns: [ { label: "First Name", dataField: "firstName", icon: "firstName" }, { label: "Last Name", dataField: "lastName", icon: "lastName" }, { label: "Birthday", dataField: "birthday", icon: "birthday", formatSettings: { formatString: "d" } }, { label: "Pet Name", dataField: "petName", icon: "petName" }, { label: "Country", dataField: "country", icon: "country" }, { label: "Product Name", dataField: "productName", icon: "productName" }, { label: "Price", dataField: "price", icon: "price", formatSettings: { formatString: "c2" } }, { label: "Quantity", dataField: "quantity", icon: "quantity", formatFunction: function(value) { let className; if (value < 20) { className = "red"; } else if (value < 35) { className = "yellow"; } else { className = "green"; } return `<div class="${className}">${value}</div>`; } }, { label: "Time of Purchase", dataField: "timeOfPurchase", icon: "timeOfPurchase", formatSettings: { formatString: "hh:mm tt" } }, { label: "Expired", dataField: "expired", icon: "expired", formatFunction: function(value) { return value ? "☑" : "☐"; } }, { label: "Attachments", dataField: "attachments" } ], coverField: "attachments", titleField: "firstName" }; } } ); } }; </script> <style> .firstName:after { background-image: url("https://img.icons8.com/ios/16/000000/user-filled.png"); } .lastName:after { background-image: url("https://img.icons8.com/ios/16/000000/signature-filled.png"); } .birthday:after { background-image: url("https://img.icons8.com/ios/16/000000/birthday-filled.png"); } .petName:after { background-image: url("https://img.icons8.com/ios/16/000000/pet-commands-follow-filled.png"); } .productName:after { background-image: url("https://img.icons8.com/ios/16/000000/box-filled.png"); } .price:after { background-image: url("https://img.icons8.com/ios/16/000000/price-tag-euro-filled.png"); } .quantity:after { background-image: url("https://img.icons8.com/ios/16/000000/negative-dynamic-filled.png"); } .country:after { background-image: url("https://img.icons8.com/ios/16/000000/country-filled.png"); } .timeOfPurchase:after { background-image: url("https://img.icons8.com/ios/16/000000/alarm-clock-filled.png"); } .expired:after { background-image: url("https://img.icons8.com/ios/16/000000/expired-filled.png"); } .red, .yellow, .green { display: inline-block; border-radius: 10px; padding: 5px; } .red { background-color: #e94f37; color: white; } .yellow { background-color: #ffee93; } .green { background-color: #63c7b2; color: white; } </style>
We can now use the smart-card-view with Vue. Data binding and event handlers will just work right out of the box.
We have bound the properties of the smart-card-view to values in our Vue component.
Running the Vue application
Start the app withnpm run devand open localhost:8080 in your favorite web browser to see the output below:
Read more about using Smart UI for Vue: https://www.htmlelements.com/docs/vue/.