Data Binding
Gantt Tasks are binded to the Smart.Gantt component as an Array of objects
.
It is possible to create complex nested strctures that illustrate a project schedule with dependency links between each individual task and their current status.
First, we need to set the TaskColumn and specify the properties of the GanttDataRecord()
object
@using System.Text.Json.Serialization; <GanttChart DataSource="Tasks" TaskColumns="taskColumns"> </GanttChart> @code{ List<GanttChartTaskColumn> taskColumns = new List<GanttChartTaskColumn>{ new GanttChartTaskColumn() { Label = "Tasks", Value = "label" }, new GanttChartTaskColumn() { Label = "Duration (hours)", Value = "duration" } }; }
Then, create an Array of GanttDataRecord()
objects:
@code{ .... List<GanttDataRecord> Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", Expanded = true, Type = "project" }, new GanttDataRecord() { Label = "Hire Employees", DateStart = "2020-4-05", DateEnd = "2021-04-10", Type = "task" }, new GanttDataRecord() { Label = "Market Website", DateStart = "2020-05-10", DateEnd = "2021-03-06", Type = "project" }, new GanttDataRecord() { Label = "Manafucture Products", DateStart = "2020-03-10", DateEnd = "2021-08-12" } }; }

Nested Projects & Tasks
Smart.Gantt allows you to form project hierarchy by nesting tasks into each other.
The parental task should be of type project
and it can contain records of type
project | task | milestone
Modify the "Develop Website" Project by adding additional Tasks, Milestones and Projects in the
Tasks
property.
List<GanttDataRecord> Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Create HTML Structure", DateStart = "2020-03-10", DateEnd = "2020-05-20", Type = "task" }, new GanttDataRecord() { Label = "Style Using CSS", DateStart = "2020-03-20", DateEnd = "2020-06-10", Type = "task" }, new GanttDataRecord() { Label = "Website Structure is complete", DateStart = "2020-06-11", Type = "milestone" }, new GanttDataRecord() { Label = "Add Products to Website", DateStart = "2020-06-11", Duration=200, Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Take Photos of Products", DateStart = "2020-06-12", DateEnd = "2020-08-20", Type = "task" }, new GanttDataRecord() { Label = "Write Description of Each Products", DateStart = "2020-05-02", DateEnd = "2020-12-01", Type = "task" }, new GanttDataRecord() { Label = "Add Product List to Main Page", DateStart = "2020-06-30", DateEnd = "2020-11-15", Type = "task" } } } } }, .... } ....
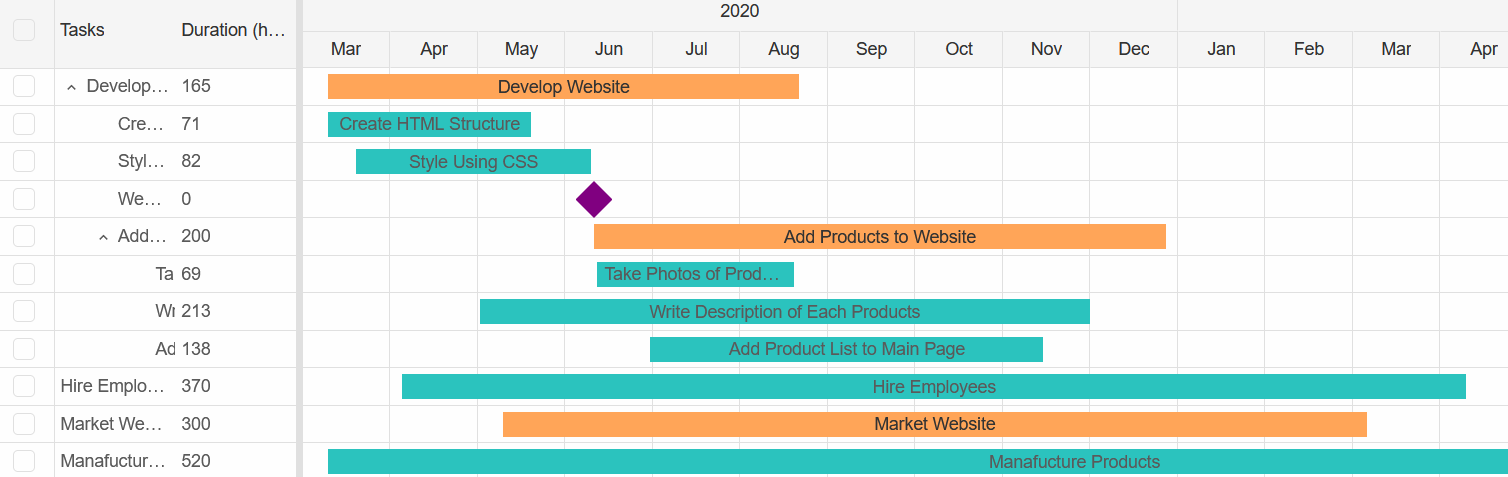
Task Connections
It is possible indicate the relation between tasks by using the Connection property.
Each connection has target
- the index of the target task,
and type
- a number representing the connection type. There are four connection types:
0
- connection of type Start-to-Start1
- connection of type End-to-Start2
- connection of type End-to-End3
- connection of type Start-to-End
Connections
property.
.... new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", Expanded = true, Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 1 }, { "type", 0 } }, new Dictionary<string, int>() { { "target", 4 }, { "type", 0 } }, new Dictionary<string, int>() { { "target", 9}, { "type", 0 } } }, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Create HTML Structure", DateStart = "2020-03-10", DateEnd = "2020-05-20", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 2 }, { "type", 2 } } } }, new GanttDataRecord() { Label = "Style Using CSS", DateStart = "2020-03-20", DateEnd = "2020-06-10", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 3 }, { "type", 0 } } } }, new GanttDataRecord() { Label = "Website Structure is complete", DateStart = "2020-06-11", Type = "milestone" }, new GanttDataRecord() { Label = "Add Products to Website", DateStart = "2020-06-11", Duration=200, Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Take Photos of Products", DateStart = "2020-06-12", DateEnd = "2020-08-20", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 6 }, { "type", 2 } } }, }, new GanttDataRecord() { Label = "Write Description of Each Products", DateStart = "2020-05-02", DateEnd = "2020-12-01", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 7 }, { "type", 2 } } }, }, new GanttDataRecord() { Label = "Add Product List to Main Page", DateStart = "2020-06-30", DateEnd = "2020-11-15", Type = "task" } } } } }, ....
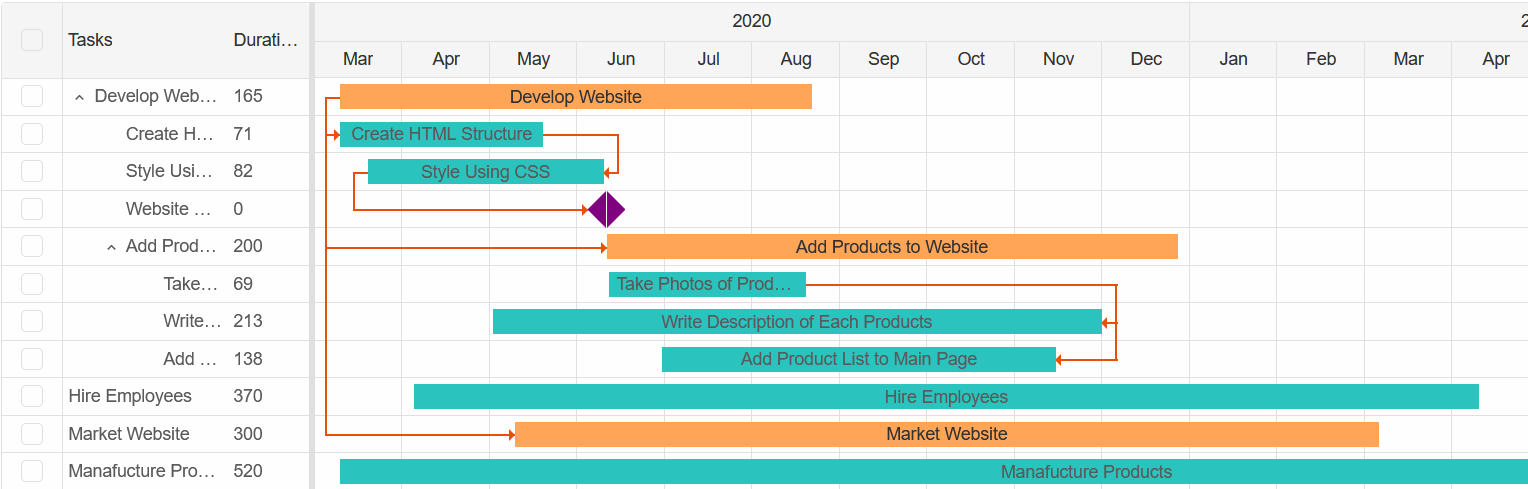
Task Progress
The Progress property allows you to set a progress value between 0 and 100.
Modify the "Hire Employees" Task by setting the progress value in the Progress
porperty.
Note that the user can change the progress of a task by selecting a task and sliding the progress thumb
.... new GanttDataRecord() { Label = "Hire Employees", DateStart = "2020-4-05", DateEnd = "2021-04-10", Type = "task", Progress=60 }, ....
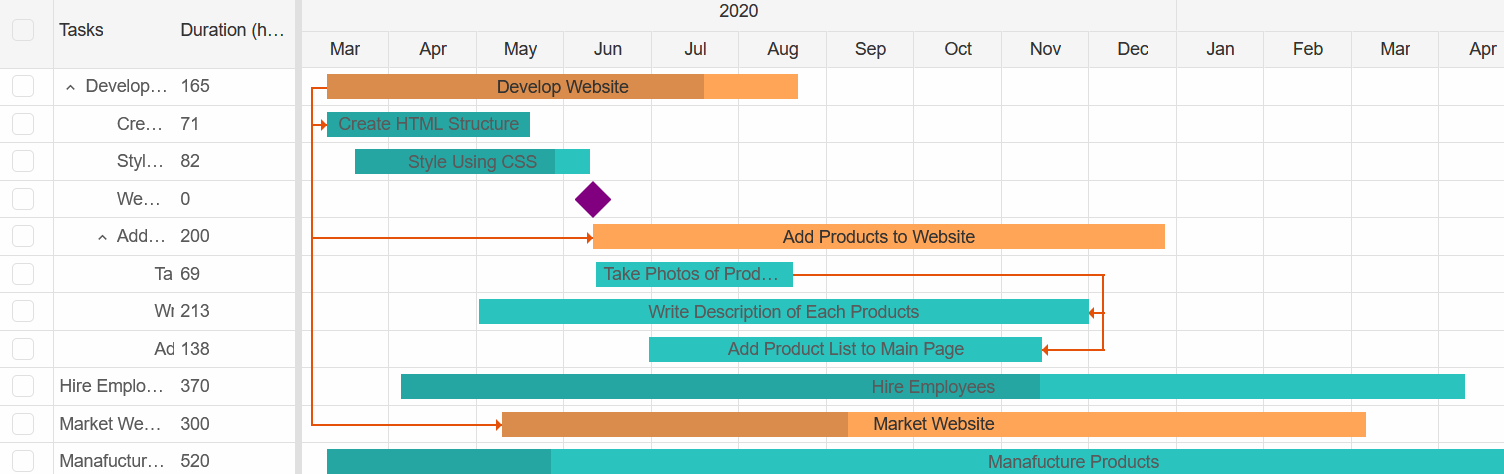
Task Duration
If you are unsure of the EndDate of a Task, it is also possible to set the Duration value.
The value will use the units as set in the DurationUnit property of the Component.
Modify the Market Website Task by replacing the EndDate
property with
Duration
value.
.... new GanttDataRecord() { Label = "Market Website", DateStart = "2020-05-10", Duration = 50, Type = "project" }, ....
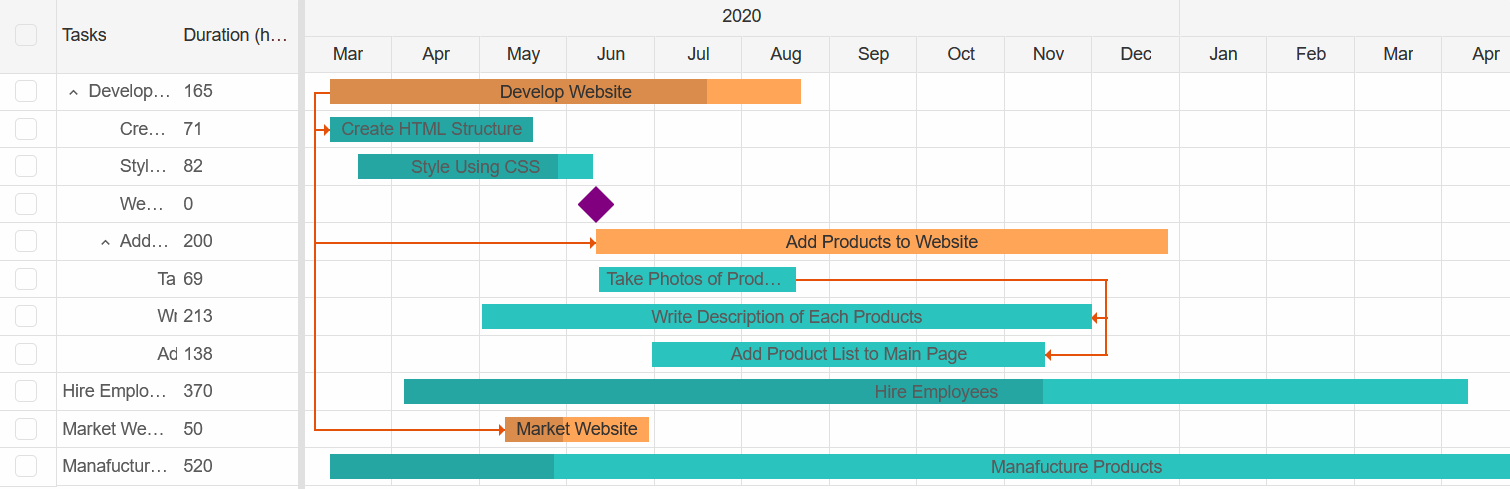
Task Properties
Each object from the DataSouce Array
required the following properties:
Label
- The label of the Task.
Acceptsstring
DateStart
- The starting date of the Task.
Acceptsstring representing a valid date
DateEnd
- The ending date of the Task.
Acceptsstring representing a valid date
Can be omitted ifDuration
is setType
- Determines the type of the Task.
Acceptstask | project | milestone
Connections
- Indicates the relation between tasks.
Acceptsarray of objects
Each object must contain:target
- The index of the taget task.number
type
- The type of the connection.0 | 1 | 2 | 3
Duration
- Determines the duration of a Task.
Acceptsnumber
- represents time units as set in theDurationUnit
property of the Component.MinDuration
- Determines the minimum duration of a Task.
Acceptsnumber
- represents time units as set in theDurationUnit
property of the Component.MaxDuration
- Determines the maximum duration of a Task.
Acceptsnumber
- represents time units as set in theDurationUnit
property of the Component.MinDateStart
- Determines the mininum date that a task can start from.
Acceptsstring representing a valid date
MaxDateStart
- Determines the maximum date that a task can start from.
Acceptsstring representing a valid date
MinDateEnd
- Determines the mininum date that a task can end.
Acceptsstring representing a valid date
MaxDateEnd
- Determines the maximum date that a task can end.
Acceptsstring representing a valid date
DisableDrag
- Disables the dragging of a task.
Acceptstrue | fasle
DisableResize
- Disables the resizing of a task.
Acceptstrue | fasle
DragProject
- Determines whether or not the whole project (along with the tasks) can be dragged while dragging the project task.
Acceptstrue | fasle
Applies only to Projects TasksSynchronized
-Determines whether the project task's start/end dates are automatically calculated based on the tasks.
Acceptstrue | fasle
Applies only to Projects TasksDisableResize
- Determines whether a project is expanded or not.
Acceptstrue | fasle
Applies only to Projects Tasks