One of the main features of the Smart.Blazor.Grid is it's features for editing its content.
The following code example showcases how to use this functionality in variuos ways.
Cells editing
'Cells editing' functionality is one of easiest to be set for the Smart.Blazor.Grid component
@using Smart.Blazor.Demos.Data @inject RandomDataService dataService <style> body, html, app { height: 100%; } app { overflow: auto; } .content { height: calc(100% - 70px); } /* This is the CSS used in the demo */ @@media only screen and (max-width: 700px) { smart-grid { width: 100%; } } </style> <h1>Grid Cells Editing</h1> <p> Click on any cell to begin edit its value. To confirm the changes, press 'Enter' or click on another cell or outside the Grid. To cancel the changes, press 'Escape'. </p> @if (dataRecords == null) { <p><em>Loading...</em></p> } else { <Grid DataSource="dataRecords" Editing="@editing"> <Columns> <Column DataField="FirstName" Label="First Name"></Column> <Column DataField="LastName" Label="Last Name"></Column> <Column DataField="ProductName" Label="Product"></Column> <Column DataField="Expired" Label="Expired" DataType="boolean" Template="@checkBoxEditor" Editor="@checkBoxEditor"></Column> <Column DataField="Quantity" Label="Quantity" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right"></Column> <Column DataField="Price" Label="Unit Price" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right" CellsFormat="c2"></Column> </Columns> </Grid> } @code { private string checkBoxEditor = "checkBox"; private string numberInputEditor = "numberInput"; GridEditing editing = new GridEditing() { Enabled = true, Mode = GridEditingMode.Cell }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(1000); } }
Setting the Grid Editing like so enables you to edit a cell from the Grid.
To begin editing of a cell simply click on a cell.
Command Column in Data Grid
One of the Data Grid editing features is the command column. The command column can be used to 'Edit' or 'Delete' a row. It can be positioned as first or last column in the Grid. The Column width is 'auto' by default, but it can be fixed as well. The 'commands' can be 'icons', 'labels' or 'icons and labels'.
The following code showcases how to set up this functionality.
<Grid DataSource="dataRecords" Appearance="@appearance" Editing="@editing"> <Columns> <Column DataField="FirstName" Label="First Name"></Column> <Column DataField="LastName" Label="Last Name"></Column> <Column DataField="ProductName" Label="Product" Editor="@autoCompleteEditor"></Column> <Column DataField="Expired" Label="Expired" DataType="boolean" Template="@checkBoxEditor" Editor="@checkBoxEditor"></Column> <Column DataField="Quantity" Label="Quantity" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right"></Column> <Column DataField="Price" Label="Unit Price" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right" CellsFormat="c2"></Column> </Columns> </Grid> @code { private string autoCompleteEditor = "autoComplete"; private string checkBoxEditor = "checkBox"; private string numberInputEditor = "numberInput"; GridAppearance appearance = new GridAppearance() { ShowRowHeaderNumber = true }; GridEditing editing = new GridEditing() { Enabled = true, Action = GridEditingAction.None, Mode = GridEditingMode.Row, CommandColumn = new GridEditingCommandColumn() { Visible = true } }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(1000); } }
This provides interactive UI for the editing.
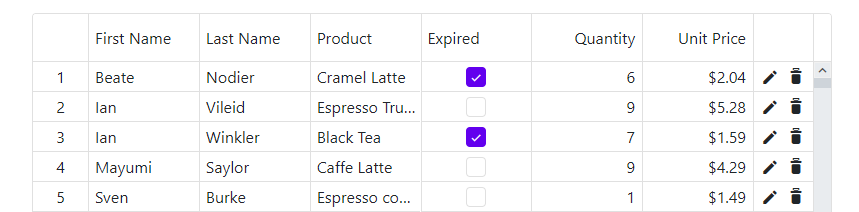
Dialog Row Editing in the Grid
Another option for Cell and Row editing the Data for the Grid component is to use a dialog.
The complete set up for this functionality is in the code below:
<Grid DataSource="dataRecords" Appearance="@appearance" Editing="@editing"> <Columns> <Column DataField="FirstName" Label="First Name"></Column> <Column DataField="LastName" Label="Last Name"></Column> <Column DataField="ProductName" Label="Product" Editor="@autoCompleteEditor"></Column> <Column DataField="Expired" Label="Expired" DataType="boolean" Template="@checkBoxEditor" Editor="@checkBoxEditor"></Column> <Column DataField="Quantity" Label="Quantity" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right"></Column> <Column DataField="Price" Label="Unit Price" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right" CellsFormat="c2"></Column> </Columns> </Grid> @code { private string autoCompleteEditor = "autoComplete"; private string checkBoxEditor = "checkBox"; private string numberInputEditor = "numberInput"; GridAppearance appearance = new GridAppearance() { ShowRowHeaderNumber = true }; GridEditing editing = new GridEditing() { Enabled = true, Mode = GridEditingMode.Row, Action = GridEditingAction.None, Dialog = new Dialog() { Enabled = true }, CommandColumn = new GridEditingCommandColumn() { Visible = true, Position = Position.Far } }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(1000); } }
When the 'Edit' icon is clicked the following modal window is displayed for editing the Row's data:
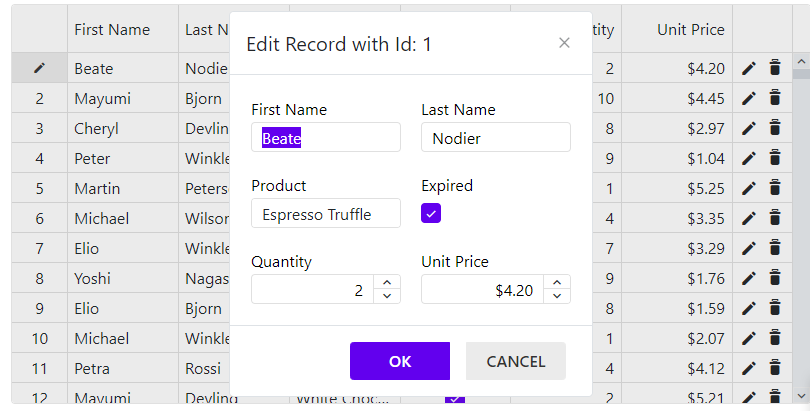
Editing validation
The 'validationRules' property determines the validation rules of the column values. The available set of rules are: 'min', 'max', 'minLength', 'maxLength', 'minDate', 'maxDate', 'null', 'email', 'required', 'requiredTrue' and 'pattern'. A cell editor is active until a valid value is entered by the user.
<Grid DataSource="dataRecords" Editing="@editing" Selection="@selection"> <Columns> <Column DataField="FirstName" Label="First Name" ValidationRules="@stringValidationRules"></Column> <Column DataField="LastName" Label="Last Name" ValidationRules="@stringValidationRules"></Column> <Column DataField="ProductName" Label="Product" ValidationRules="@stringValidationRules"></Column> <Column DataField="Expired" Label="Expired" DataType="boolean" Template="@checkBoxEditor" Editor="@checkBoxEditor" ValidationRules="@boolValidationRules"></Column> <Column DataField="Quantity" Label="Quantity" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right" ValidationRules="@numberValidationRules"> </Column> <Column DataField="Price" Label="Unit Price" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right" CellsFormat="c2" ValidationRules="@numberValidationRules"> </Column> </Columns> </Grid> } @code { private string checkBoxEditor = "checkBox"; private string numberInputEditor = "numberInput"; GridEditing editing = new GridEditing() { Enabled = true, Mode = GridEditingMode.Row }; GridSelection selection = new GridSelection() { Enabled = true, AllowCellSelection = true, AllowRowHeaderSelection = true, AllowColumnHeaderSelection = true, Mode = GridSelectionMode.Extended }; IList<IDictionary<string, object>> stringValidationRules = new List<IDictionary<string, object>>() { new Dictionary<string, object>() { { "type", "required" } }, new Dictionary<string, object>() { { "type", "minLength"}, { "value", 5 } } }; IList<IDictionary<string, object>> boolValidationRules = new List<IDictionary<string, object>>() { new Dictionary<string, object>() { { "type", "requiredTrue" } } }; IList<IDictionary<string, object>> numberValidationRules = new List<IDictionary<string, object>>() { new Dictionary<string, object>() { { "type", "min" }, { "value", 1 } }, new Dictionary<string, object>() { { "type", "max"}, { "value", 20 } } }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(100); } }
If the data entered doesn't meet the validation requirements this how the Grid will be displayed:
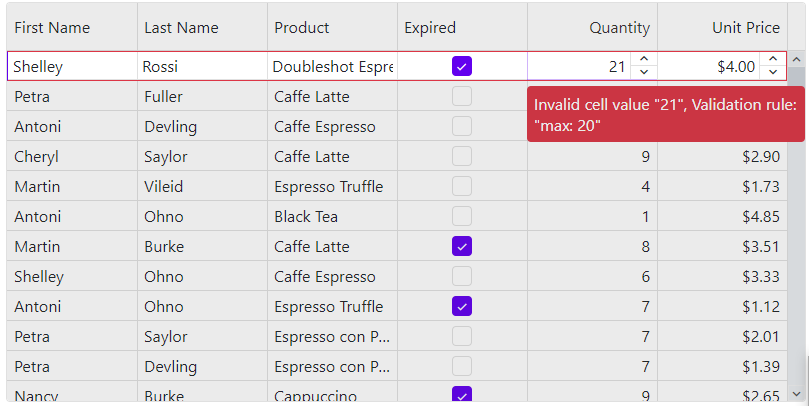
Batch Editing
The data grid allows you to edit and update multiple grid rows on the client side and send them with a single server request. To enable the Grid's batch editing, the 'editing.batch' property should be set to 'true' The 'editing.commandBar' setting allows you to display a toolbar or statusbar with tools for saving and reverting the edit made by the users. This is done by setting 'editing.commandBar.visible' to 'true'.
Code set up for the Grid
<Grid DataSource="dataRecords" Appearance="@appearance" Editing="@editing"> <Columns> <Column DataField="FirstName" Label="First Name"></Column> <Column DataField="LastName" Label="Last Name"></Column> <Column DataField="ProductName" Label="Product" Editor="@autoCompleteEditor"></Column> <Column DataField="Expired" DataType="boolean" Label="Expired" Template="@checkBoxEditor" Editor="@checkBoxEditor"></Column> <Column DataField="Quantity" Label="Quantity" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right"></Column> <Column DataField="Price" Label="Unit Price" DataType="number" Editor="@numberInputEditor" Align="HorizontalAlignment.Right" CellsAlign="HorizontalAlignment.Right" CellsFormat="c2"></Column> </Columns> </Grid> @code { private string autoCompleteEditor = "autoComplete"; private string checkBoxEditor = "checkBox"; private string numberInputEditor = "numberInput"; GridAppearance appearance = new GridAppearance() { ShowRowHeaderNumber = true }; GridEditing editing = new GridEditing() { Batch = true, Enabled = true, Action = GridEditingAction.Click, AddDialog = new Dialog() { Enabled = true }, CommandBar = new GridEditingCommandBar() { Visible = true, Position = LayoutPosition.Far } }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(1000); } }