Blazor - Get Started with Smart.Tree
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Tree
Smart.Tree represents a nested list of selectable items.
- Add the Tree component to the Pages/Index.razor file
<Tree></Tree>
- Inside the
@code
block, create an array of elements and set it as DataSource of the component<Tree DataSource="items"></Tree> @code{ string[] items = new string[]{"Affogato", "Americano", "Bicerin", "Breve" }
- Alternatively, set the items as children of the component. Item can be expandede by default with
IsExpanded
:<Tree> <TreeItemsGroup IsExpanded>Drinks <TreeItemsGroup IsExpanded> Juices <TreeItem Selected>Apple Juice</TreeItem> <TreeItem>Orange Juice</TreeItem> <TreeItem>Lemon Juice</TreeItem> </TreeItemsGroup> <TreeItemsGroup> Coffee <TreeItem>Affogato</TreeItem> <TreeItem>Americano</TreeItem> <TreeItem>Bicerin</TreeItem> </TreeItemsGroup> </TreeItemsGroup> <TreeItemsGroup>Food <TreeItem>Pizza</TreeItem> <TreeItem>Burger</TreeItem> <TreeItem>Sushi</TreeItem> </TreeItemsGroup> </Tree>
-
Defult selection can be achieved by adding the
Selected
property to a TreeItem:<TreeItem Value="1" Selected>Affogato</TreeItem>
Or by using the
SelectedIndexes
orSelectedValues
properties:<Tree SelectedIndexes="@indexes"> .... </Tree> @code{ string[] indexes = new string[]{0}; }
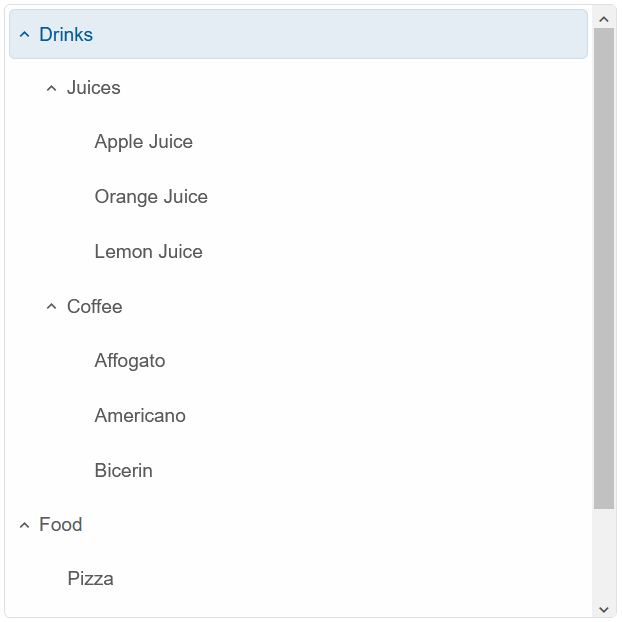
Selection Modes
The selection mode of the component can be set to nine different modes:
CheckBox | One | None | OneOrMany | OneOrManyExtended | RadioButton | ZeroAndOne | ZeroOrMany | ZeroOrOne
The selection mode is set to Checkbox:
<Tree DataSource="@items" SelectionMode="TreeSelectionMode.CheckBox"></Tree>
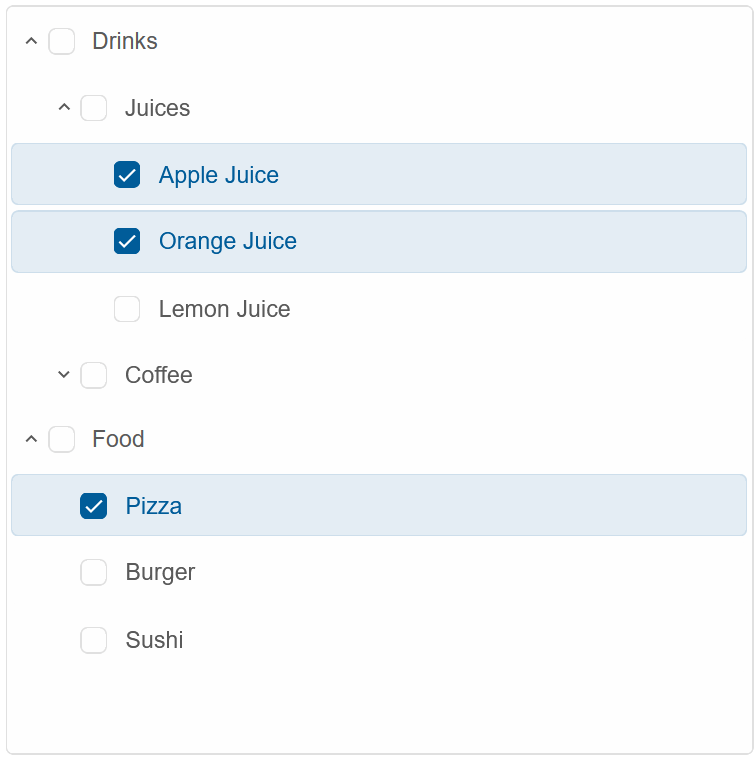
Filtering
Smart.Tree's built-in filtering functionality can be enabled using the Filterable
property.
In addition, FilterMode
determines the filtering rules such as contains, equals,startsWith and many more.
FilterMember
determines which TreeItem property will be used as filtering criteria. By default it is the label
<Tree DataSource="@items" Filterable FilterMode="FilterMode.Contains" FilterInputPlaceholder="Search for item..."></Tree>
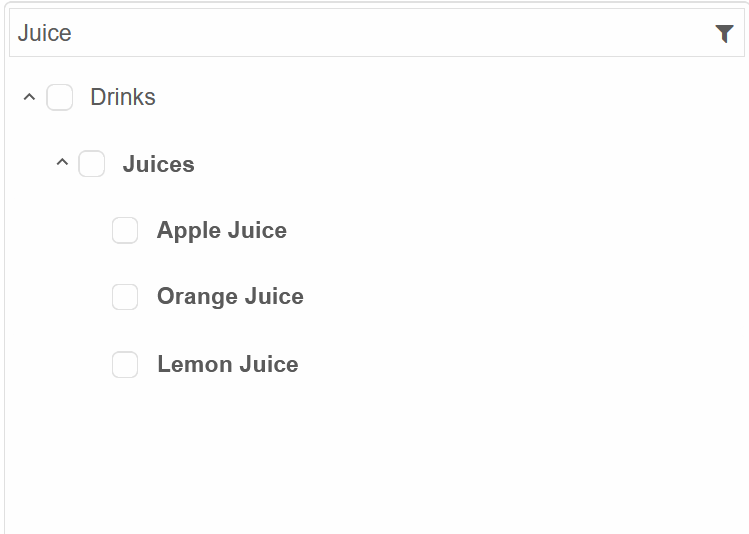
Sorting
The Tree can also be sorted in a ascending or descending order or with a custom sorting function:
<Tree DataSource="@items" Sorted="true" SortDirection="TreeSortDirection.Asc"></Tree>
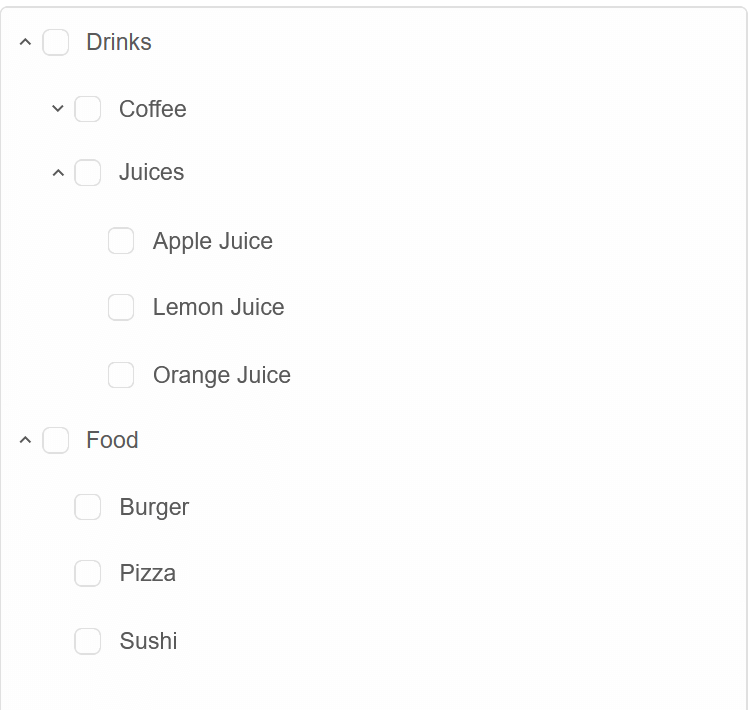
Drag & Drop
The AllowDrag
and AllowDrop
properties allow you to drag items from one group to another.
They can also be dragged to a different Tree elements:
<Tree DataSource="@items" AllowDrag AllowDrop></Tree>
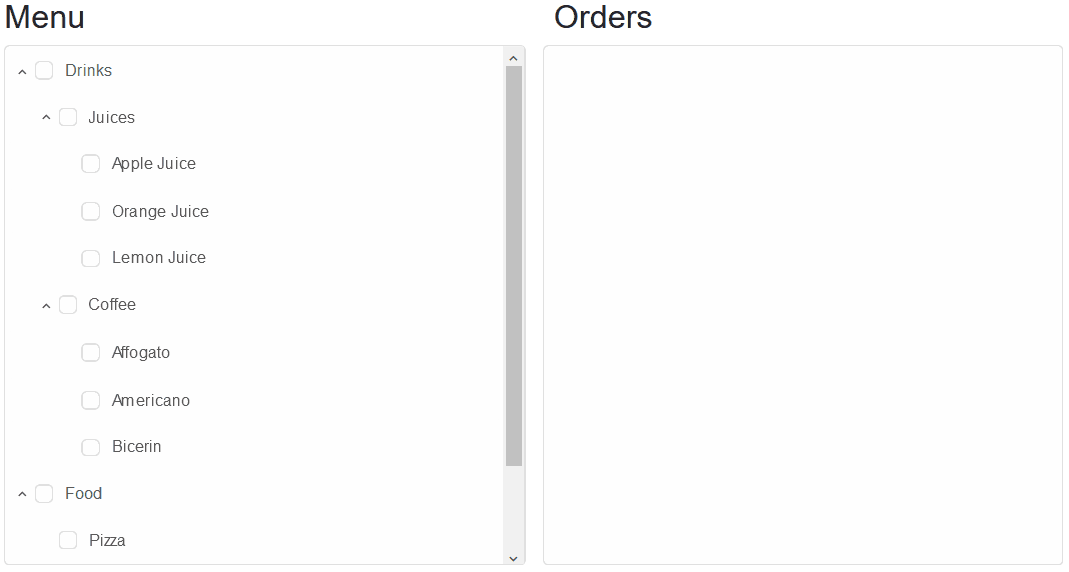
Tree Events
Smart.Tree provides multiple Events that can help you expand the component's functionality.
Each event object has unique event.detail parameters.
OnChange
- triggered when the selection is changed.
Event Details
: dynamic item, IEnumerableoldSelectedIndexes, IEnumerable selectedIndexes OnCollapse
- triggered when a jqx-tree-items-group is collapsed.
Event Details
: dynamic item, string label, dynamic path, dynamic value, dynamic childrenOnCollapsing
- triggered when a jqx-tree-items-group is about to be collapsed.
Event Details
: dynamic item, string label, dynamic path, dynamic value, dynamic childrenOnDragEnd
- This event is triggered when a jqx-tree-item/jqx-tree-items-group is dropped somewhere in the DOM.
Event Details
: dynamic container, dynamic data, dynamic item, dynamic items, dynamic originalEvent, dynamic previousContainer, dynamic targetOnDragging
- triggered when a jqx-tree-item/jqx-tree-items-group is being dragged.
Event Details
: dynamic data, dynamic item, dynamic items, dynamic originalEventOnDragStart
- triggered when a dragging operation is started in jqx-tree.
Event Details
: dynamic container, dynamic data, dynamic item, dynamic items, dynamic originalEvent, dynamic previousContainerOnExpand
- triggered when a jqx-tree-items-group is expanded.
Event Details
: dynamic item, string label, dynamic path, dynamic value, dynamic childrenOnExpanding
- triggered when a jqx-tree-items-group is about to be expanded.
Event Details
: dynamic item, string label, dynamic path, dynamic value, dynamic childrenOnScrollBottomReached
- triggered when the Tree has been scrolled to the bottom.
Event Details
: N/AOnScrollTopReached
- triggered when the Tree has been scrolled to the top.
Event Details
: N/AOnSwipeleft
- triggered when the user swipes to the left inside the Tree.
Event Details
: N/AOnSwiperight
- triggered when the user swipes to the right inside the Tree..
Event Details
: N/A
The demo below uses the OnChange Event to create a menu where users can select one item from each category, then the category is disabled:
<Tree SelectionMode="TreeSelectionMode.CheckBox" OnChange="OnChange"> <TreeItemsGroup IsExpanded>Drinks <TreeItemsGroup @ref="@juices" IsExpanded> Juices <TreeItem>Apple Juice</TreeItem> <TreeItem>Orange Juice</TreeItem> <TreeItem>Lemon Juice</TreeItem> </TreeItemsGroup> <TreeItemsGroup @ref="@coffee" IsExpanded> Coffee <TreeItem>Affogato</TreeItem> <TreeItem>Americano</TreeItem> <TreeItem>Bicerin</TreeItem> </TreeItemsGroup> </TreeItemsGroup> <TreeItemsGroup @ref="@food" IsExpanded>Food <TreeItem>Pizza</TreeItem> <TreeItem>Burger</TreeItem> <TreeItem>Sushi</TreeItem> </TreeItemsGroup> </Tree> @code{ string selection = ""; TreeItemsGroup juices, coffee, food; private void OnChange(Event ev){ TreeChangeEventDetail detail = ev["Detail"]; foreach(string item in detail.SelectedIndexes){ if(item.StartsWith("0.0")){ juices.IsExpanded = false; juices.Disabled = true; } else if(item.StartsWith("0.1")){ coffee.IsExpanded = false; coffee.Disabled = true; } else if(item.StartsWith("1")){ food.IsExpanded = false; food.Disabled = true; } } } }
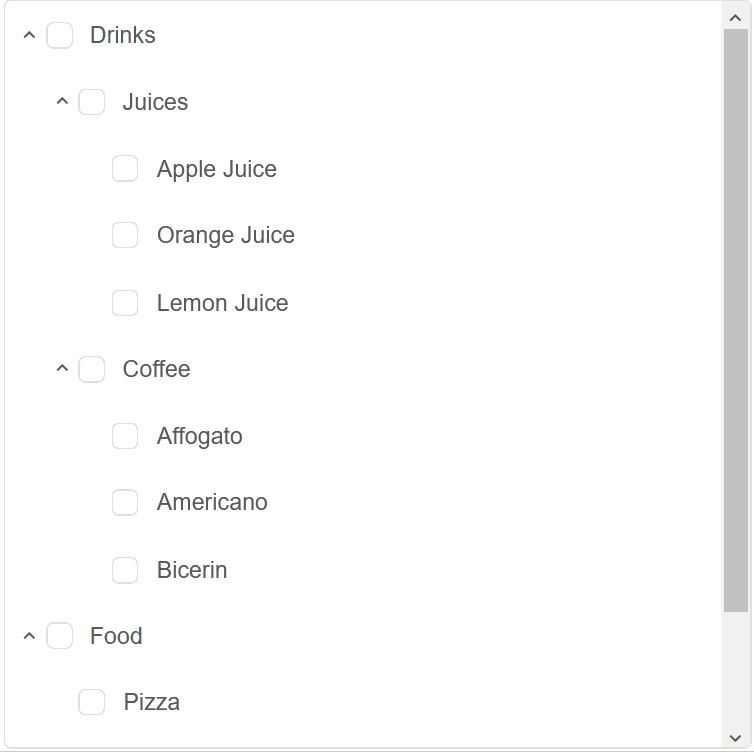