Using with Vue 3 and TypeScript
In this tutorial we will show you how to use Smart Web Components with Vue and TypeScript.
We will show examples of DropDownList and Grid.
Set Up Project
First, make sure you have the latest version of Node.js and npm installed.
Then you need to intall vue-cli if you haven't already. You can do it with the following command:
npm install @vue/cli
Once the cli is installed navigate to the folder where you want to create your application and run the following command:
vue create my-app
The cli will let you choose the configuration settings.
Firstly, select the option for manually selecting features:
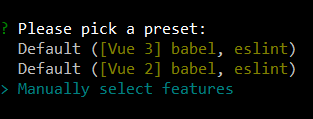
Then select the TypeScript option checkbox:
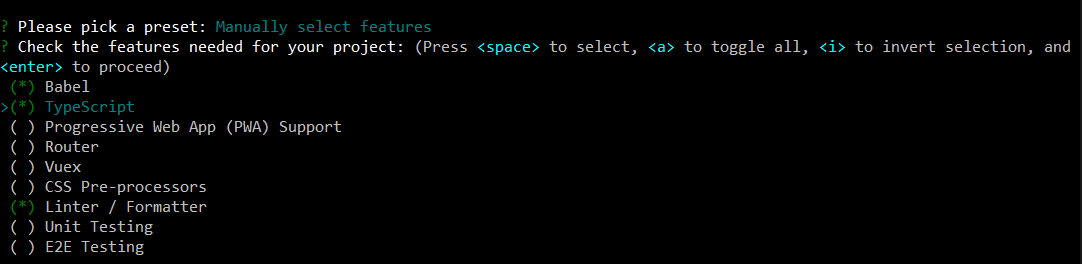
And finally choose version 3 of Vue:
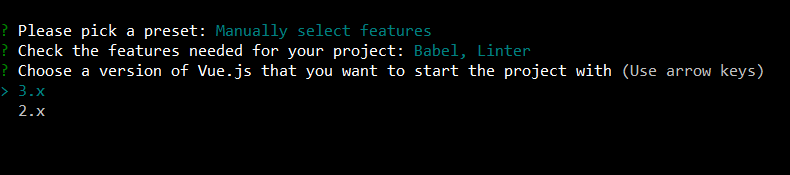
You can choose the rest of the setting depending on your preferences. This will create your new Vue project.
Install Smart Web Components
Next we need to install Smart Web Components.
Open my-app folder in you command prompt and run:
npm install smart-webcomponents
Import Styles
After installing the package we are ready to start using the components.
Open the App.vue file and import the smart.default.css file, like this:
import 'smart-webcomponents/source/styles/smart.default.css';
Use Smart DropDownList for Vue with TypeScript
After that import the smart.dropdownlist.js file and the DropDownListProperties interface from the drop down list typings file so we can use the property types:
import "smart-webcomponents/source/modules/smart.dropdownlist.js"; import { DropDownListProperties } from "smart-webcomponents/source/typescript/smart.dropdownlist"
Change the component template to the following:
<template> <div class="vue-root"> <smart-drop-down-list ref="dropDownList1" selected-indexes="selectedIndexes"> <smart-list-item value="1">Affogato</smart-list-item> <smart-list-item value="2">Americano</smart-list-item> <smart-list-item value="3">Bicerin</smart-list-item> <smart-list-item value="4">Breve</smart-list-item> <smart-list-item value="5">Cappuccino</smart-list-item> <smart-list-item value="6">Cafe Crema</smart-list-item> <smart-list-item value="7">Cafe Corretto</smart-list-item> <smart-list-item value="8">Cafe macchiato</smart-list-item> <smart-list-item value="9">Cafe mocha</smart-list-item> <smart-list-item value="10">Cortado</smart-list-item> <smart-list-item value="11">Cuban espresso</smart-list-item> <smart-list-item value="12">Espresso</smart-list-item> <smart-list-item value="13">Eiskaffee</smart-list-item> <smart-list-item value="14">Frappuccino</smart-list-item> <smart-list-item value="15">Galao</smart-list-item> <smart-list-item value="16">Greek frappe coffee</smart-list-item> <smart-list-item value="17">Iced Coffee</smart-list-item> <smart-list-item value="18">Instant Coffee</smart-list-item> <smart-list-item value="19">Latte</smart-list-item> <smart-list-item value="20">Liqueur coffee</smart-list-item> </smart-drop-down-list> </div> </template>
Next, define the selectedIndexes of type DropDownListProperties["selectedIndexes"] in the data section, like this:
data:() => { const selectedIndexes: DropDownListProperties["selectedIndexes"] = [0]; return { selectedIndexes } }
And finally, assign them to the DropDownList component inside the mounted lifecycle hook:
mounted: function() { (this.$refs.dropDownList1 as DropDownList).selectedIndexes = this.selectedIndexes; }
Here is how the full App.vue looks now:
<template> <div class="vue-root"> <smart-drop-down-list ref="dropDownList1"> <smart-list-item value="1">Affogato</smart-list-item> <smart-list-item value="2">Americano</smart-list-item> <smart-list-item value="3">Bicerin</smart-list-item> <smart-list-item value="4">Breve</smart-list-item> <smart-list-item value="5">Cappuccino</smart-list-item> <smart-list-item value="6">Cafe Crema</smart-list-item> <smart-list-item value="7">Cafe Corretto</smart-list-item> <smart-list-item value="8">Cafe macchiato</smart-list-item> <smart-list-item value="9">Cafe mocha</smart-list-item> <smart-list-item value="10">Cortado</smart-list-item> <smart-list-item value="11">Cuban espresso</smart-list-item> <smart-list-item value="12">Espresso</smart-list-item> <smart-list-item value="13">Eiskaffee</smart-list-item> <smart-list-item value="14">Frappuccino</smart-list-item> <smart-list-item value="15">Galao</smart-list-item> <smart-list-item value="16">Greek frappe coffee</smart-list-item> <smart-list-item value="17">Iced Coffee</smart-list-item> <smart-list-item value="18">Instant Coffee</smart-list-item> <smart-list-item value="19">Latte</smart-list-item> <smart-list-item value="20">Liqueur coffee</smart-list-item> </smart-drop-down-list> </div> </template> <script lang="ts"> import { defineComponent } from 'vue'; import 'smart-webcomponents/source/styles/smart.default.css'; import "smart-webcomponents/source/modules/smart.dropdownlist.js"; import { DropDownList, DropDownListProperties } from "smart-webcomponents/source/typescript/smart.dropdownlist" export default defineComponent({ name: 'App', data:() => { const selectedIndexes: DropDownListProperties["selectedIndexes"] = [0]; return { selectedIndexes } }, mounted: function() { (this.$refs.dropDownList1 as DropDownList).selectedIndexes = this.selectedIndexes; } }); </script>
When you run the app using npm run serve you get the following result:
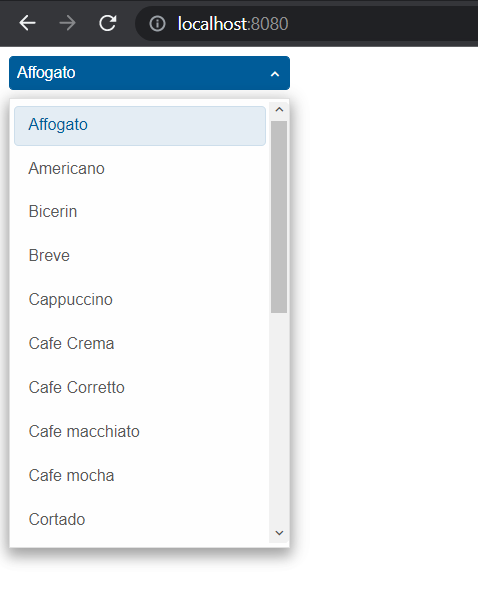
Use Smart Grid for Vue with TypeScript
Here is an example of how you can use Smart.Grid for Vue with TypeScript:
<template> <smart-grid id="grid"></smart-grid> </template> <script lang="ts"> import { defineComponent } from 'vue'; import 'smart-webcomponents/source/styles/smart.default.css'; import "smart-webcomponents/source/modules/smart.grid.js"; import { GridProperties, GridDataSourceSettings } from "smart-webcomponents/source/typescript/smart.grid" export default defineComponent({ name: 'App', components: { }, mounted: function() { const columns: GridProperties["columns"] = [ { label: 'First Name', dataField: 'firstName' }, { label: 'Last Name', dataField: 'lastName' }, { label: 'Product', dataField: 'productName' } ]; const dataFields: GridDataSourceSettings["dataFields"] = [ { name: 'firstName', dataType: 'string' }, { name: 'lastName', dataType: 'string' }, { name: 'productName', dataType: 'string' } ]; const dataSource: GridProperties["dataSource"] = new window.Smart.DataAdapter( { dataSource: [ { "firstName": "Beate", "lastName": "Wilson", "productName": "Caramel Latte"}, { "firstName": "Ian", "lastName": "Nodier", "productName": "Caramel Latte"}, { "firstName": "Petra", "lastName": "Vileid", "productName": "Green Tea"}, { "firstName": "Mayumi", "lastName": "Ohno", "productName": "Caramel Latte"}, { "firstName": "Mayumi", "lastName": "Saylor", "productName": "Espresso con Panna"}, { "firstName": "Regina", "lastName": "Fuller", "productName": "Caffe Americano" }, { "firstName": "Regina", "lastName": "Burke", "productName": "Caramel Latte"}, { "firstName": "Andrew", "lastName": "Petersen", "productName": "Caffe Americano"}, { "firstName": "Martin", "lastName": "Ohno", "productName": "Espresso con Panna"}, { "firstName": "Beate", "lastName": "Devling", "productName": "Green Tea"}, { "firstName": "Sven", "lastName": "Devling", "productName": "Espresso Truffle"}, { "firstName": "Petra", "lastName": "Burke", "productName": "Peppermint Mocha Twist"}, { "firstName": "Marco", "lastName": "Johnes", "productName": "Caffe Mocha"} ], dataFields }); window.Smart("#grid", class { get properties() { return { columns, dataSource } } }); } }); </script>
When you run the app using npm run serve you get the following result:
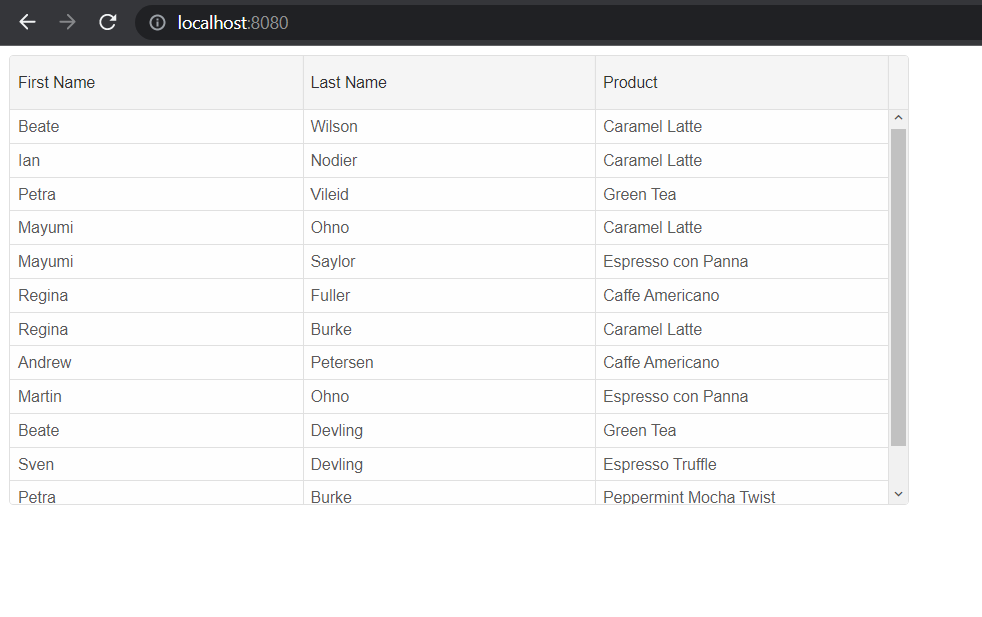