Bind IEnumerable to Blazor Smart.Grid
Setup The Blazor Application
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Bind IEnumerable Array to Grid
For the purpose of the Demo, we will use the built-in WeatherForecast Service
inside the Data
folder.
Add the Grid component and Grid Columns to the Pages/Index.razor
file.
The Date Column can be easily formatted using the CellsFormat property.
<Grid DataSource="@forecast" DataSourceSettings="@dataSourceSettings"> <Columns> <Column DataField="Date" Label="Date" DataType="date" CellsFormat="M" Width="200"> </Column> <Column DataField="TemperatureC" Label="Temp. (C)" Width="200"> </Column> <Column DataField="TemperatureF" Label="Temp. (F)" Width="150"> </Column> <Column DataField="Summary" Label="Summary"> </Column> </Columns> </Grid>
Inside the @code
block, set the DataSource
property of the Grid to the Array data
from the WeatherService.
@code{ private WeatherForecast[] forecast; protected override async Task OnInitializedAsync() { forecast = await ForecastService.GetForecastAsync(DateTime.Now); } }Then specify the DataSourceType inside a
GridDataSourceSettings
object and set it as a
property of the Grid.Note that setting the DataType of the Columns is not mandatory, but it is recommended if you plan to use the Smart.Grid's Filtering & Sorting functionalities
<Grid DataSource="@forecast" DataSourceSettings="@dataSourceSettings"> ... </Grid> @code { .... GridDataSourceSettings dataSourceSettings = new GridDataSourceSettings() { DataFields = new List<IGridDataSourceSettingsDataField>() { new GridDataSourceSettingsDataField() { Name = "Date", DataType = GridDataSourceSettingsDataFieldDataType.Date }, new GridDataSourceSettingsDataField() { Name = "TemperatureC", DataType = GridDataSourceSettingsDataFieldDataType.Number }, new GridDataSourceSettingsDataField() { Name = "TemperatureF", DataType = GridDataSourceSettingsDataFieldDataType.Number }, new GridDataSourceSettingsDataField() { Name = "Summary", DataType = GridDataSourceSettingsDataFieldDataType.String }, }, DataSourceType = GridDataSourceSettingsDataSourceType.Array }; }
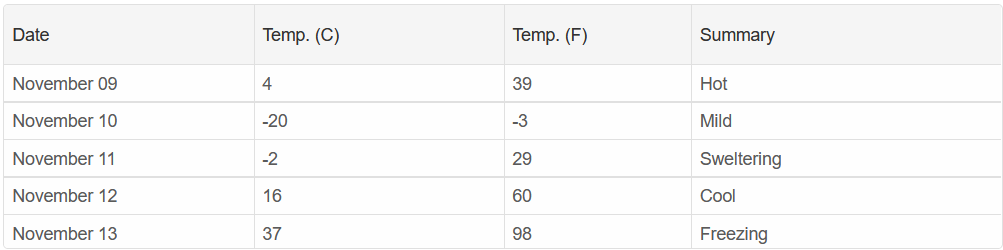
Continue from here
Follow the Get Started with Grid guide to learn more about many of the features offered by Blazor Smart.Grid component.
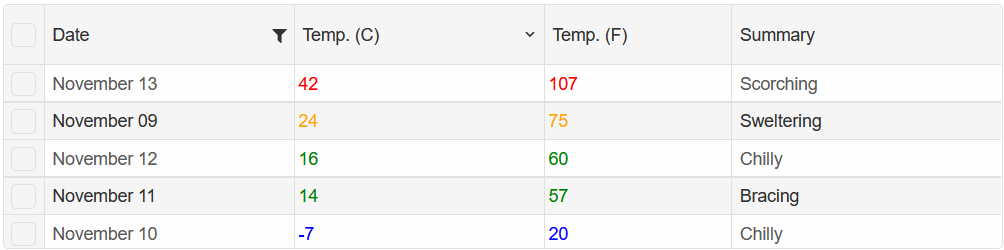