Blazor - Get Started with Smart.NumberInput
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Number Input
Smart.NumberInput is a custom number input element with additional built-in features such as a number formatting and styling.
- Add the NumberInput component to the Pages/Index.razor file
<NumberInput></NumberInput>
- Inside the component, set additional properties such as Min and Max values:
<NumberInput Min="0" Max="10" Value="5"></NumberInput>

Number Formatting
Smart.NumberInput implements
Intl.NumberFormat API
for number formatting.
Some of the possible number formats are decimal
, currency
, percent
and unit
<NumberInput Min="0" Max="10" Value="5" NumberFormat="currencyFormat"></NumberInput> <NumberInput Min="0" Max="10" Value="5" NumberFormat="customFormat"></NumberInput> @code{ private object currencyFormat = new { style = "currency", currency = "USD" }; private object customFormat = new { style = "unit", unit = "liter", unitDisplay= "long" }; }
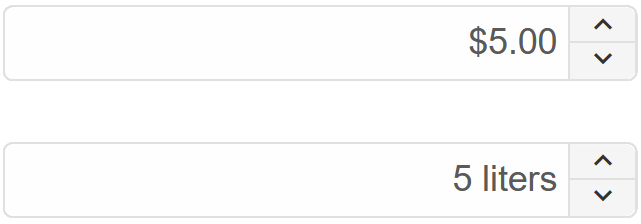
NumberInput Customization
Smart.NumberInput can be styled in different ways by setting the Class property.
The NumberInput can be default
, outlined
or underlined
<NumberInput Min="0" Max="10" Value="5" NumberFormat="currencyFormat" Class="underlined"></NumberInput>
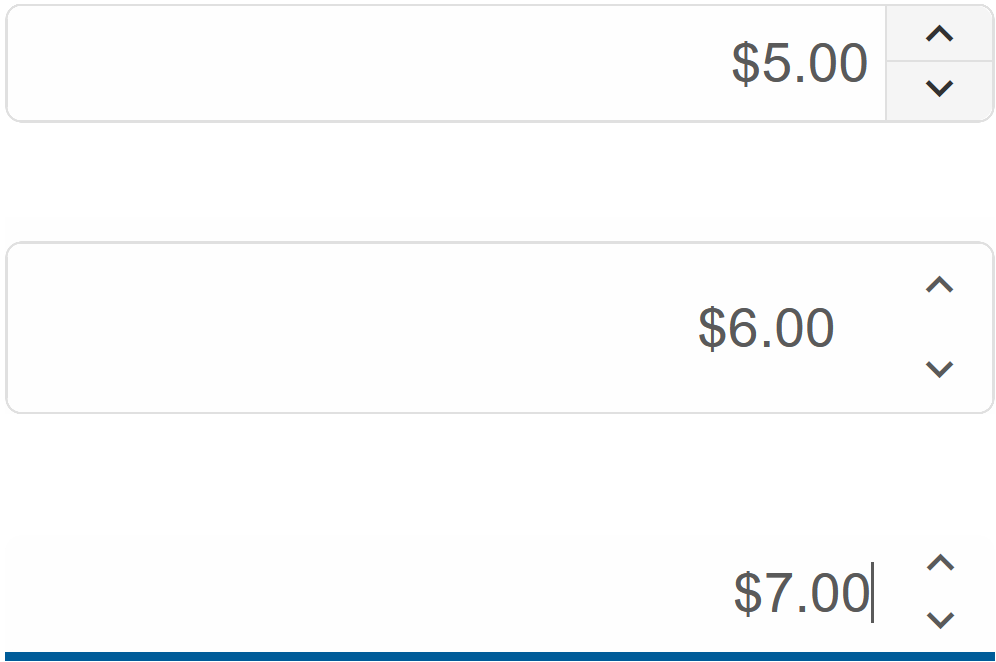
NumberInput Events
Smart.NumberInput provides an OnChange Event that can help you expand the component's functionality.
The event object has unique event.detail parameters.
OnChange
- triggered when the selection is changed.
Event Details
: string label, dynamic oldLabel, dynamic oldValue, dynamic value
The demo below uses the OnChange Event to sum all previous values of the NumberInput:
<NumberInput NumberFormat="currencyFormat" Class="outlined" OnChange="OnChange"></NumberInput> <h2>Some of all values: @sum</h2> @code{ int sum = 0; private void OnChange(Event ev) { if(ev.ContainsKey("Detail")){ NumberInputChangeEventDetail detail = ev["Detail"]; sum+= detail.Value; } } }
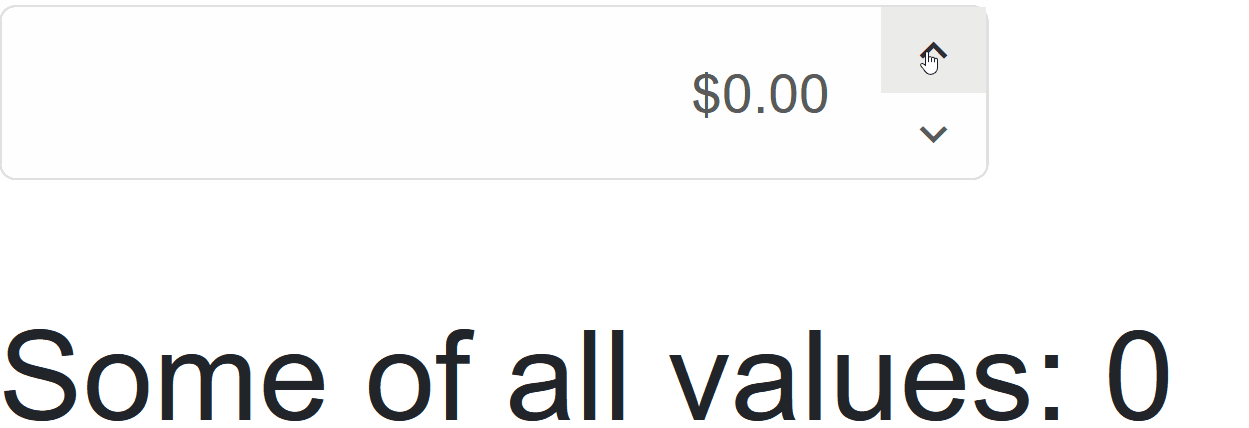