Angular Grid with Data Service
Setup The Angular Application
Follow the Getting Started guide to set up your Angular application with Smart UI.
Setup the Angular Smart.Grid Component
Follow the Angular Grid guide to read more about how you can use the Angular Grid component.
Data Service
In this guide we will show you how to bind Angular Smart Grid to a data service.
First, we will create a simple DataService with a single method called getData
that will return an Observable
with a data array:
import { Injectable } from '@angular/core'; import { Observable, of } from 'rxjs'; import { GetData } from '../assets/data'; @Injectable({ providedIn: 'root', }) export class DataService { constructor() { } getData(): Observable{ const data = of(GetData(15)); return data; } }
The service uses GetData
method from assets/data.ts file that looks like this:
interface IRowGenerateData { id: number; reportsTo: number | null; available: boolean | null; firstName: string; lastName: string; name: string; productName: string; quantity: string | number; total: string | number; price: string | number; date: Date; leaf: boolean; } export function GetData(rowscount?: number, last?: number, hasNullValues?: boolean): IRowGenerateData[] { const data: IRowGenerateData[] = new Array(); if (rowscount === undefined) { rowscount = 100; } let startIndex = 0; if (last) { startIndex = rowscount; rowscount = last - rowscount; } const firstNames = [ 'Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene' ]; const lastNames = [ 'Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier' ]; const productNames = [ 'Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Caramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist' ]; const priceValues = [ '2.25', '1.5', '3.0', '3.3', '4.5', '3.6', '3.8', '2.5', '5.0', '1.75', '3.25', '4.0' ]; for (let i = 0; i < rowscount; i++) { const row = {} as IRowGenerateData; const productindex = Math.floor(Math.random() * productNames.length); const price = parseFloat(priceValues[productindex]); const quantity = 1 + Math.round(Math.random() * 10); row.id = startIndex + i; row.reportsTo = Math.floor(Math.random() * firstNames.length); if (i % Math.floor(Math.random() * firstNames.length) === 0) { row.reportsTo = null; } row.available = productindex % 2 === 0; if (hasNullValues === true) { if (productindex % 2 !== 0) { const random = Math.floor(Math.random() * rowscount); row.available = i % random === 0 ? null : false; } } row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.name = row.firstName + ' ' + row.lastName; row.productName = productNames[productindex]; row.price = price; row.quantity = quantity; row.total = price * quantity; const date = new Date(); date.setFullYear(2016, Math.floor(Math.random() * 11), Math.floor(Math.random() * 27)); date.setHours(0, 0, 0, 0); row.date = date; data[i] = row; } return data; }
Then, inside app.component.ts we will inject our data service in the constuctor and in the OnInit
lifecycle hook we will subscribe to the getData
observable of the service and will update the dataSource
:
import { Component, OnInit } from '@angular/core'; import { GridColumn, Smart } from 'smart-webcomponents-angular/grid'; import { DataService } from 'src/services/data-service'; @Component({ selector: 'app-root', templateUrl: './app.component.html' }) export class AppComponent implements OnInit { constructor(private dataService: DataService) {} dataSource: any[] = []; columns: GridColumn[] = [ { label: 'First Name', dataField: 'firstName' }, { label: 'Last Name', dataField: 'lastName' }, { label: 'Product', dataField: 'productName' }, { label: 'Quantity', dataField: 'quantity' }, { label: 'Unit Price', dataField: 'price', cellsFormat: 'c2' }, { label: 'Total', dataField: 'total', cellsFormat: 'c2' } ]; ngOnInit(): void { this.dataService.getData() .subscribe(data => this.dataSource = data); } }Result:
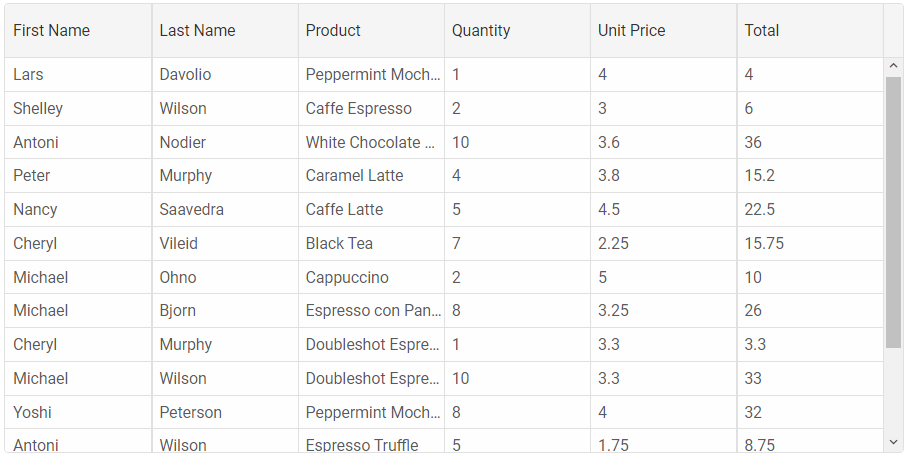