Task Customization
Smart.Gantt offers a wide variety of Task properties, which allow you to fully customize the tasks and display more information to the user.
Task Baseline
Task Baseline is an immovable line under a task. It can be used to indicate the planned duration of a given task.
It is set using the Planned
property:
<style> .smart-gantt-chart { height: auto; --smart-gantt-chart-task-default-height: 50px; } </style> <h2> Gantt Chart </h2> <GanttChart DataSource="@Records" DurationUnit="Duration.Hour" ShowBaseline="true"/> @code { public partial class GanttDataRecord { [JsonPropertyName("label")] public string Label { get; set; } [JsonPropertyName("dateStart")] public string DateStart { get; set; } [JsonPropertyName("dateEnd")] public string DateEnd { get; set; } [JsonPropertyName("type")] public string Type { get; set; } [JsonPropertyName("duration")] public int Duration { get; set; } [JsonPropertyName("tooltip")] public string Tooltip { get; set; } [JsonPropertyName("deadline")] public string Deadline { get; set; } [JsonPropertyName("planned")] public object Planned { get; set; } = new object[] {}; [JsonPropertyName("segments")] public object Segments { get; set; } = new object[] {}; } public List<GanttDataRecord> Records = new List<GanttDataRecord>() { new GanttDataRecord{ Label = "Develop Website", DateStart = "2021-01-01", DateEnd = "2021-01-20", Type = "task", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-01" }, { "dateEnd", "2021-01-20" } } }, new GanttDataRecord{ Label = "Marketing Campaign", DateStart = "2021-01-05", DateEnd = "2021-01-15", Type = "task", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-051" }, { "dateEnd", "2021-01-15" } } }, new GanttDataRecord{ Label = "Publishing", DateStart = "2021-01-10", DateEnd = "2021-01-26", Type = "task", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-10" }, { "dateEnd", "2021-01-26" } } }, new GanttDataRecord{ Label = "Find clients", DateStart = "2021-01-12", DateEnd = "2021-01-25", Type = "task", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-12" }, { "dateEnd", "2021-01-25" } } } }; }
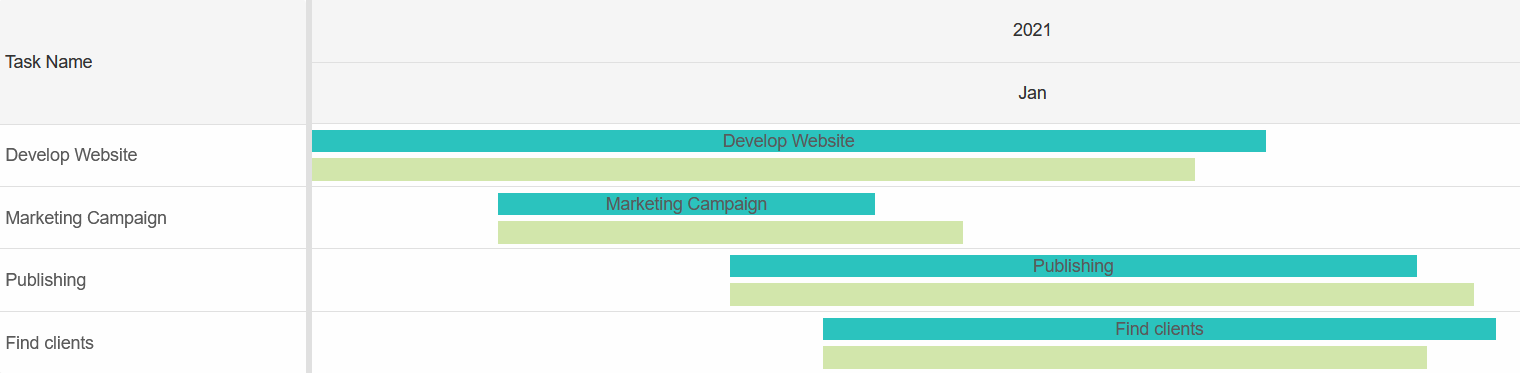
Task Deadline
Task Deadline is a red marker, which indicated the deadline of a given task.
When the Task is past the deadline, Smart.Gantt will automatically display the overdue time of the task.
The overdue units are set according to the DurationUnit
property (default is Milisecond
)
Modify the first Task by including a Deadline
property:
.... new GanttDataRecord{ Label = "Develop Website", DateStart = "2021-01-01", DateEnd = "2021-01-20", Type = "task", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-01" }, { "dateEnd", "2021-01-20" } }, Deadline = "2021-01-21" }, ....
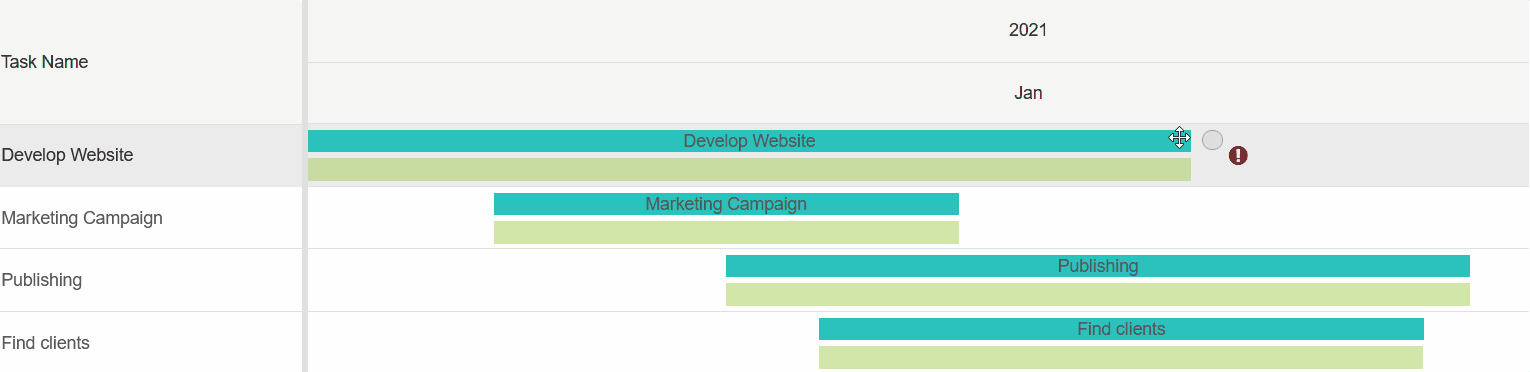
Task Segments
Tasks can be broken up into multiple segments. They can be used to indicate multiple Subtasks that together form a bigger Task.
Segments are set inside the Segments
property. Modify the "Marketing Campaign" by creating multiple segments:
..... new GanttDataRecord{ Label = "Marketing Campaign", DateStart = "2021-01-05", DateEnd = "2021-01-20", Type = "task", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-051" }, { "dateEnd", "2021-01-20" } }, Segments = new List<Dictionary<string, string>>() { new Dictionary<string, string>() { { "label", "Facebook Marketing" }, { "dateStart", "2021-01-05" }, { "dateEnd", "2021-01-10" } }, new Dictionary<string, string>() { { "label", "Instagram Marketing" }, { "dateStart", "2021-01-11" }, { "dateEnd", "2021-01-16" } }, new Dictionary<string, string>() { { "label", "Brochures" }, { "dateStart", "2021-01-17" }, { "dateEnd", "2021-01-20" } }, } }, ....
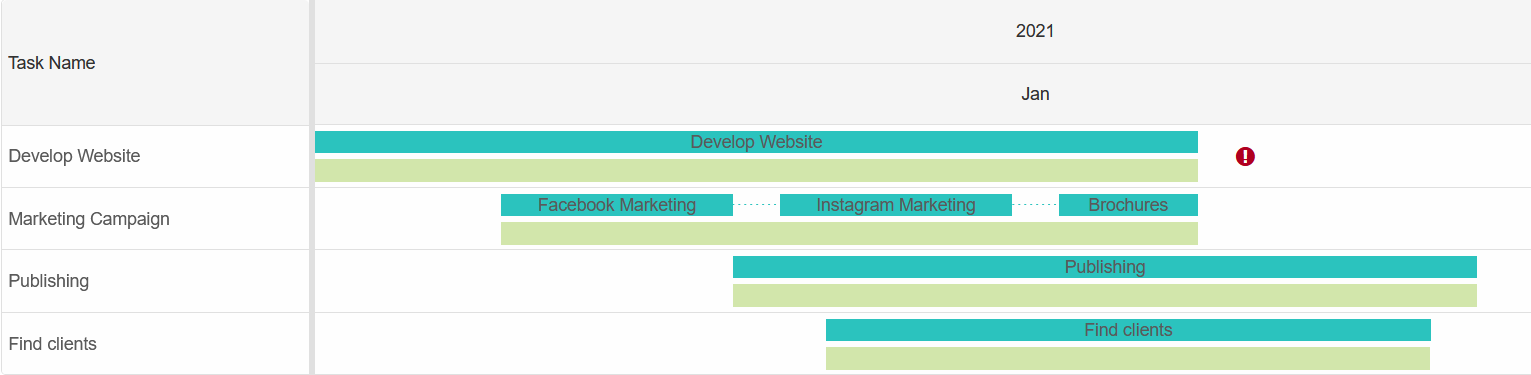
Task Tooltips
Task Tooltips allow you to diplay additional information about a given task.
First, Tooltips need to be enabled inside the GanttChartTooltip
object.
All Tasks will display a default tooltip, including Task Label, Date Start and Date End.
The tooltip of each Task can be modified inside the Tooltip
property:
<GanttChart DataSource="@Records" DurationUnit="Duration.Hour" ShowBaseline="true" Tooltip="tooltip"/> @code{ .... public GanttChartTooltip tooltip = new GanttChartTooltip{ Enabled = true }; .... public List<GanttDataRecord> Records = new List<GanttDataRecord>() { .... new GanttDataRecord{ Label = "Publishing", DateStart = "2021-01-10", DateEnd = "2021-01-26", Type = "task", Tooltip = "<b>Find & contact a publisher</b>", Planned = new Dictionary < string, string > () { { "dateStart", "2021-01-10" }, { "dateEnd", "2021-01-26" } } }, .... } }
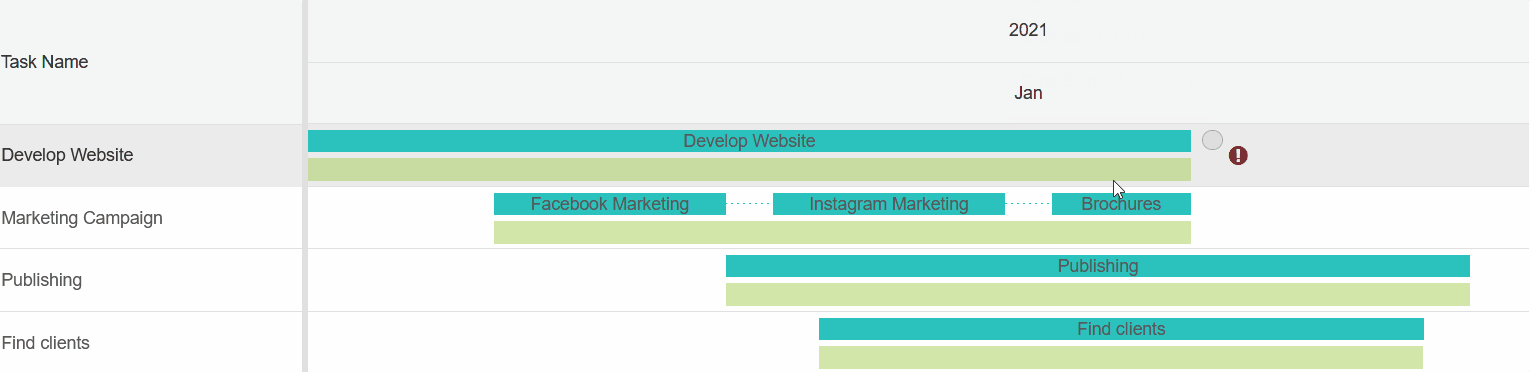