Blazor - Get Started with Smart.Tank
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Tank
Smart.Tank is a rectangular component, which allows users to select a value within a range by dragging the filled area in the component.
- Add the Tank component to the Pages/Index.razor file:
<Tank></Tank>
- Set the
Value
property to an integer value<Tank Value="50"></Tank>
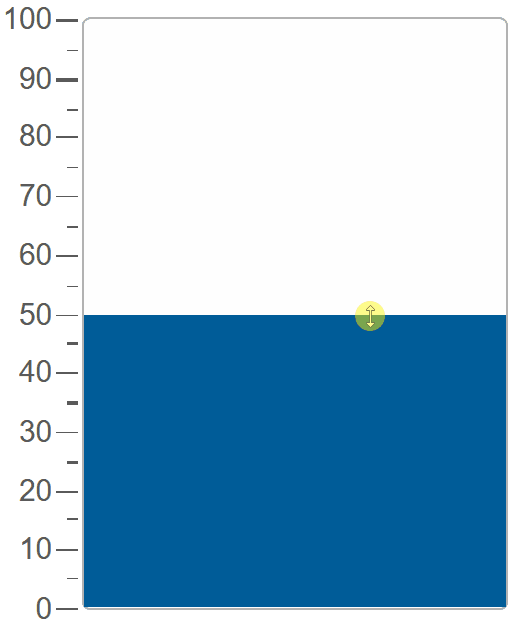
Horizontal Tank
The tank component can be set to either Verical(default)
or Horizontal
<Tank Value="50" Orientation="Orientation.Horizontal"></Tank>
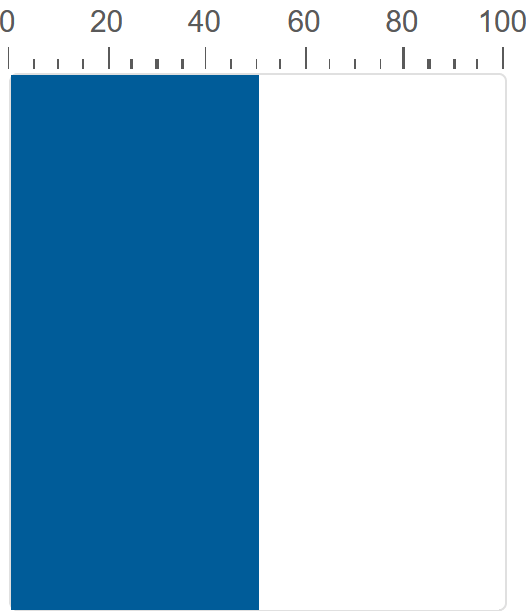
Tank Tooltip
TThe built-in tooltip feature allows users to easily see the precise value of their selection.
It is enabled with the ShowTooltip
property.
<Tank Value="50" ShowTooltip TooltipPosition="Position.Far"></Tank>
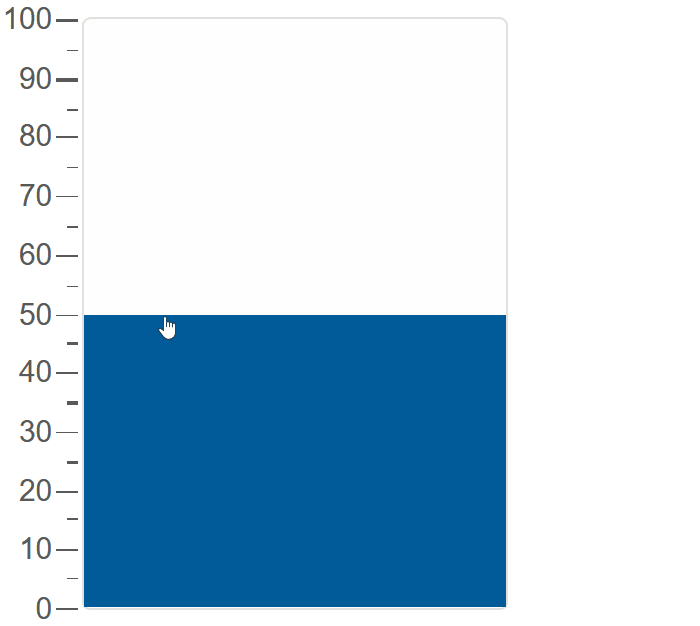
Snapping Interval
By setting the Coerce
property, the Tank will snap to the nearest value that the interval allows.
The interval between allowed values is set with the Interval
property
<Tank Value="50" Coerce Interval="10" ShowTooltip TooltipPosition="Position.Far"></Tank>
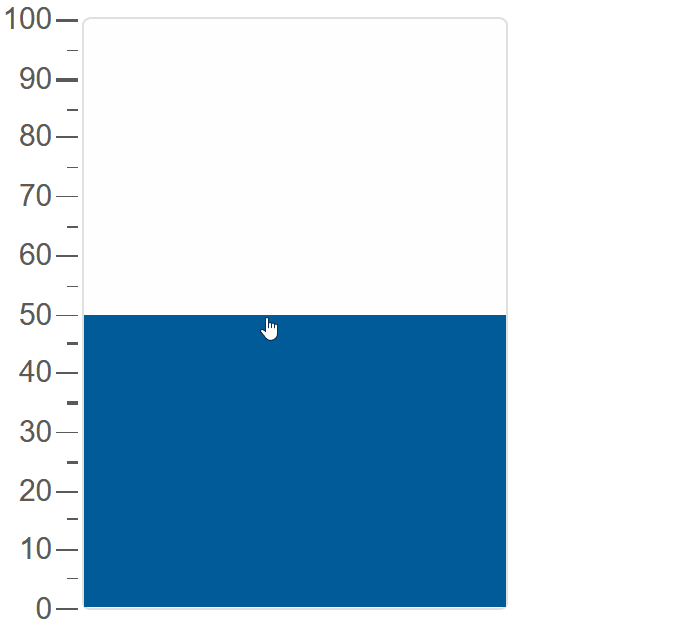
Custom Interval
The ticks of the Tank can also be set to a custom, possibly uneven interval.
The ticks are set as an array to CustomTicks
.
<Tank Value="50" ShowTooltip TooltipPosition="Position.Far" Coerce CustomTicks="@ticks" CustomInterval></Tank> @code{ int[] ticks = new int[]{1, 25, 50, 60, 100}; }
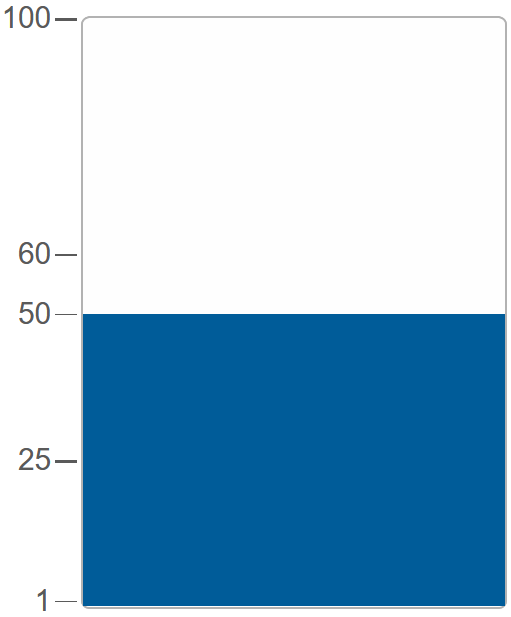
Logarithmic Tank
In addition to normal scale, Smart.Tank can be set to logarithmic mode by setting the LogarithmicScale
property.
<Tank Max="10000" ShowTooltip TooltipPosition="Position.Far" Coerce LogarithmicScale></Tank>
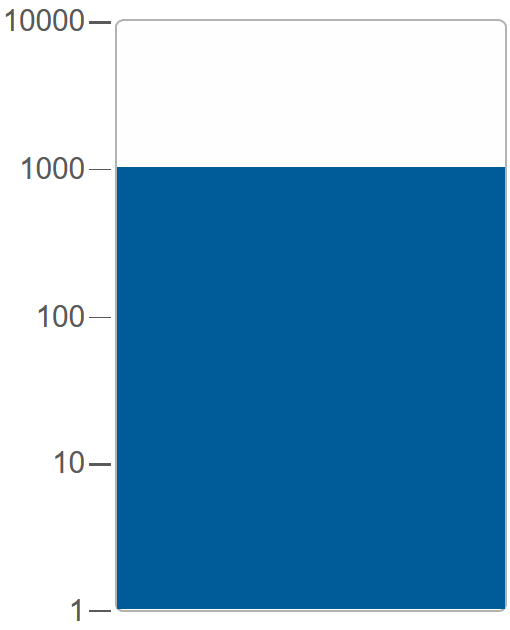
Date Tank
Smart.Tank can also create date intervals by setting the Mode
property to ScaleMode.Date
and setting Min
and Max
values.
<Tank Max="@max" Min="@min" Mode="ScaleMode.Date" ShowTooltip TooltipPosition="Position.Far"></Tank> @code{ string max = "01/01/2023"; string min = "01/01/2022"; }
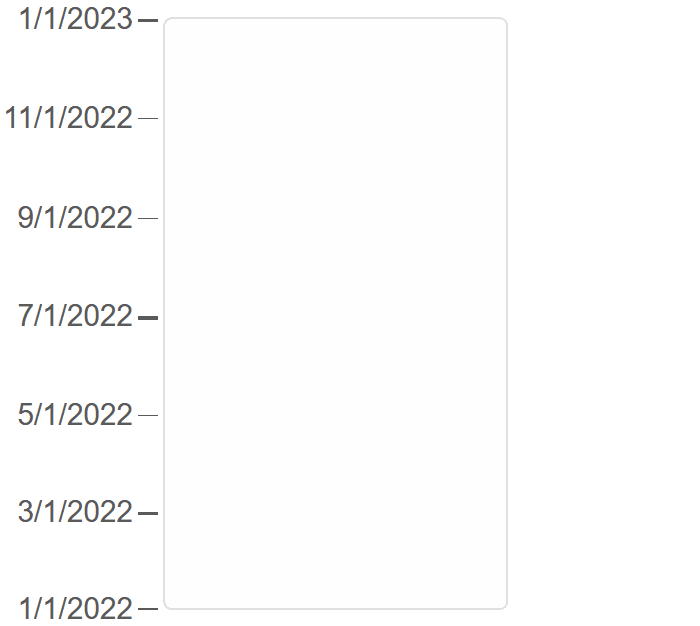
Tank Units
It is possible to display custom units next to the labels
by using the Unit
property and setting ShowUnit
<Tank Unit="gal" ShowUnit ShowTooltip TooltipPosition="Position.Far"></Tank>
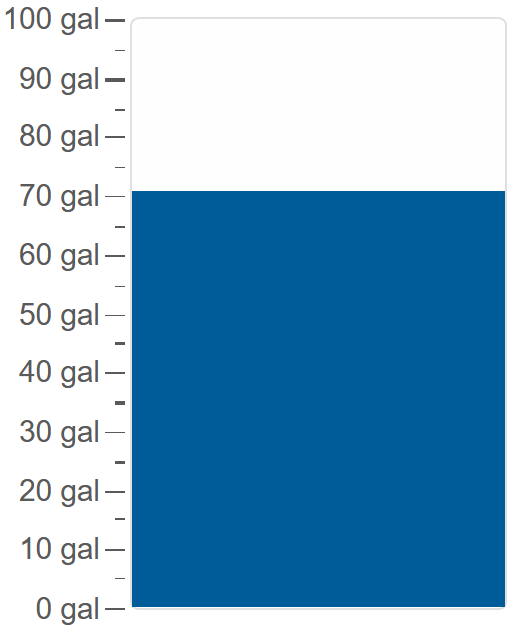
Tank Events
Smart.Tank provides an OnChange event that can help you expand the components' functionality.
OnChange
- triggered when the value of the Tank is changed.
Event Details
: dynamic value, dynamic oldValue
The demo below uses the OnChange
Event to disable the element when value becomes greater than 50:
<Tank @ref="@tank" ShowTooltip TooltipPosition="Position.Far" OnChange="OnChange" Coerce></Tank> @code{ Tank tank; private async void OnChange(Event ev){ Console.WriteLine(await tank.Val()); if(Int32.Parse(await tank.Val())>50){ tank.Disabled = true; } } }
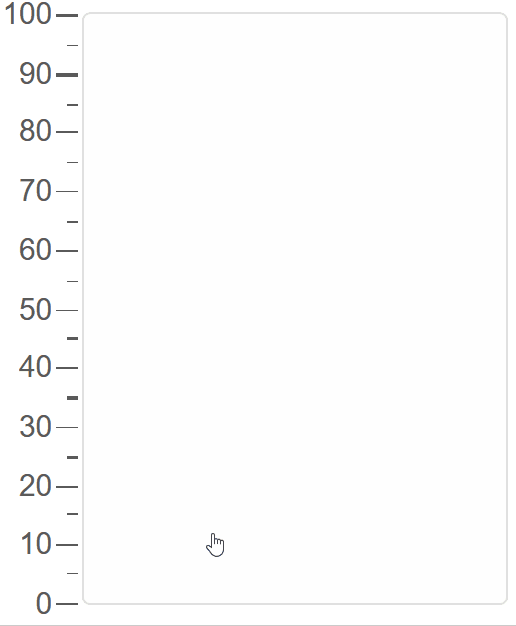