Chart Styling
Build your web apps using Smart UI
Smart.Chart - styling configuration and usage
Chart Styling
Smart.Chart offers many options to customize the chart appearance and colors. In this section we will go through the styling options available in different chart elements.
Themes
Smart.Chart themes are sets of background, line, text and band colors that will be used in the Chart by default, if no further customizations are made. There are two themes - 'light' (default) and 'dark' that can be applied by setting the theme property.
The following images show the same Chart with 'light' and 'dark' theme respectively:
![]() |
|
![]() |
|
theme: 'light' | theme: 'dark' |
Color Schemes
Smart.Chart also ships with 32 built-in color schemes which are used to automatically set colors for different series. You can change the color scheme by setting the colorScheme property of the chart. The available value are from 'scheme01' to 'scheme32'.
The following images show the same Chart with two different color schemes applied:
![]() |
|
![]() |
|
colorScheme: 'scheme28' | colorScheme: 'scheme29' |
Custom color schemes can also be applied by adding them with the addColorScheme method:
const chart = document.querySelector('smart-chart'); chart.addColorScheme('myScheme', ['#1A6642', '#46C26F', '#F9B956', '#F38443', '#DE513D']); chart.colorScheme = 'myScheme';
Custom Series Colors
Series colors can be furter customized via the following series properties:
- fillColor - fill color for the serie.
- fillColorSelected - fill color for the serie when selected.
- fillColorAlt - alternating fill color for the serie. Applicable to OHLC series only.
- fillColorAltSelected - alternating fill color for the serie when selected. Applicable to OHLC series only.
- fillColorSymbol - fill color for the marker symbols in the serie.
- fillColorSymbolSelected - fill color for the the marker symbols in serie when selected.
- legendFillColor - fill color of the legend box. The default value is inherited from the serie's color.
- legendLineColor - line color of the legend box. The default value is inherited from the serie's color.
- lineColor - line color for the serie.
- lineColorSelected - line color for the serie when selected.
- lineColorSymbol - line color for the marker symbols in serie.
- lineColorSymbolSelected - line color for the marker symbols in the serie when selected.
- toolTipLineColor - determines the tooltip's border lines color.
- useGradientColors - determines whether to use color gradients.
Conditional colors can also be applied by implementing the callback function colorFunction.
In the following example, some of these settings are demonstrated:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Custom Series Colors Example</title> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/webcomponentsjs/2.2.7/webcomponents-bundle.js"></script> <script type="text/javascript" src="../../source/smart.elements.js"></script> <script> const sampleData = [ { Day: 'Monday', Keith: 30, Erica: 15, Average: 25, Best: 60 }, { Day: 'Tuesday', Keith: 25, Erica: 25, Average: 30, Best: 100 }, { Day: 'Wednesday', Keith: 30, Erica: 20, Average: 25, Best: 170 }, { Day: 'Thursday', Keith: 35, Erica: 25, Average: 45, Best: 50 }, { Day: 'Friday', Keith: 20, Erica: 20, Average: 25, Best: 245 }, { Day: 'Saturday', Keith: 30, Erica: 20, Average: 30, Best: 295 }, { Day: 'Sunday', Keith: 60, Erica: 45, Average: 90, Best: 270 } ]; Smart('#chart', class { get properties() { return { caption: 'Fitness & exercise weekly scorecard', description: 'Time spent in vigorous exercise', showLegend: true, padding: { left: 5, top: 5, right: 5, bottom: 5 }, titlePadding: { left: 90, top: 0, right: 0, bottom: 10 }, dataSource: sampleData, xAxis: { dataField: 'Day', gridLines: { visible: true }, valuesOnTicks: false }, valueAxis: { description: 'Time in minutes', axisSize: 'auto', tickMarksColor: '#888888' }, seriesGroups: [ { type: 'column', series: [ { dataField: 'Keith', displayText: 'Keith', fillColor: '#048BA8' }, { dataField: 'Erica', displayText: 'Erica', fillColor: '#16DB93' }, { dataField: 'Average', displayText: 'Class average', useGradientColors: true, colorFunction: function (value, index) { if (isNaN(value)) { return '#C6E2E9'; } return index < 5 ? '#C6E2E9' : '#A7BED3'; } } ] }, { type: 'stackedline', series: [ { dataField: 'Best', displayText: 'All-time best', symbolType: 'circle', symbolSize: 10, fillColorSymbol: '#56013D', lineColor: '#A4036F' } ] } ] } } }); </script> </head> <body> <smart-chart id="chart"></smart-chart> </body> </html>
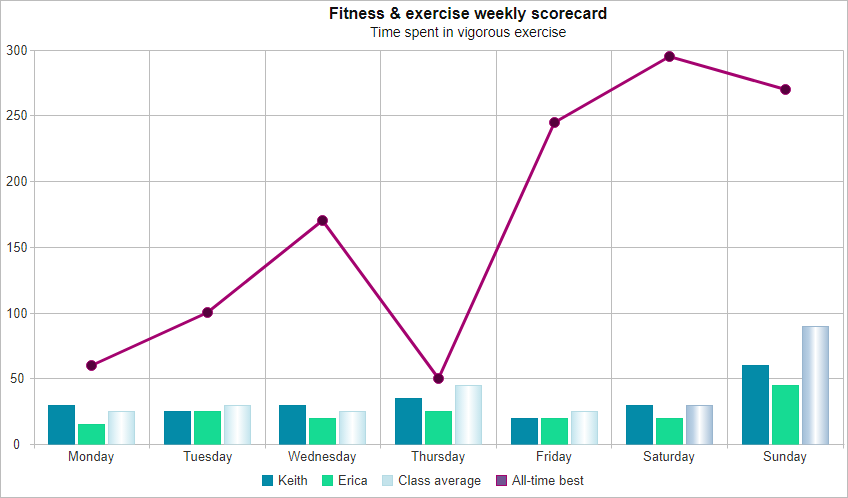
Grid lines
You can adjust the spacing and colors of the horizontal and vertical grid lines. The vertical gridlines can be adjusted by changing the xAxis.gridLines options. For example the following code makes the vertical grid lines visible, makes their color blue and sets the spacing between the lines such that a line appears once for every tenth element:
xAxis: { gridLines: { visible: true, color: '#0000FF', step: 10 } }
The horizontal grid lines can be changed by setting the valueAxis.gridLines options.
Tick marks
Tick marks and grid lines work in a very similar way and Smart.Chart offers identical properties.
To change the tick marks across the horizontal axis use the xAxis.tickMarks options:
xAxis: { tickMarks: { visible: true, color: '#0000FF', step: 2 } }
The valueAxis.tickMarks options control the appearance of the value axis tick marks.
Border Line Color, Background Color, and Background Image
You can change the chart's border color by setting the borderLineColor property:
const chart = document.querySelector('smart-chart'); chart.borderLineColor = '#0000FF';
The chart's default background color can be changed by setting the backgroundColor property:
const chart = document.querySelector('smart-chart'); chart.backgroundColor = '#97D1E5';
Instead of using background color you can use an image by setting backgroundImage:
const chart = document.querySelector('smart-chart'); chart.backgroundImage = '../images/chart_background.png';
Styling Through CSS
Some parts of Smart.Chart can be styled through CSS. Here is an overview:
CSS variables for Chart styling:
- --smart-font-family - font-family of text in the Chart.
- --smart-font-size - font-size of caption text. The font-size of all other text parts is also relative to this variable.
- --smart-border-radius - border-radius of the Chart's tooltip.
The following CSS selectors can be used to style Smart.Chart:
-
smart-chart - applied to the whole Chart element.
-
.smart-container - applied to the container that holds all internal elements of the Chart.
- .smart-chart-title-text - applied to the Chart's caption (title).
- .smart-chart-title-description - applied to the Chart's description.
- .smart-chart-axis-description - applied to axis descriptions.
- .smart-chart-axis-text - applied to axis labels.
- .smart-chart-label-text - applied to series labels.
- .smart-chart-legend-text - applied to legend text.
- .smart-chart-annotation-text - applied to annotation text.
- .smart-chart-range-selector - applied to the Chart's range selector.
-
.smart-chart-tooltip - applied to the Chart's tooltip.
-
.smart-chart-tooltip-content - applied to the tooltip's content container.
- .smart-chart-tooltip-text - applied to the tooltip's text.
-
.smart-chart-tooltip-content - applied to the tooltip's content container.
-
.smart-container - applied to the container that holds all internal elements of the Chart.
Live Example
The following live example demonstrates many of the functionalities described in this tutorial:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Custom Styling Example</title> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <style type="text/css"> #chart .smart-chart-axis-text, #chart .smart-chart-legend-text { fill: #FFFFFF; text-shadow: 3px 3px 0 #000, -1px -1px 0 #000, 1px -1px 0 #000, -1px 1px 0 #000, 1px 1px 0 #000; } .smart-chart-tooltip-text { color: #000000; } .smart-chart-tooltip-content { border-width: 2px; background: repeating-linear-gradient( 45deg, transparent, transparent 10px, #ccc 10px, #ccc 20px ), linear-gradient( to bottom, #eee, #999 ); } </style> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/webcomponentsjs/2.2.7/webcomponents-bundle.js"></script> <script type="text/javascript" src="../../source/smart.elements.js"></script> <script> const sampleData = [ { Day: 'Monday', 'Oklahoma City': 37, Sofia: 8, Bruges: 18 }, { Day: 'Tuesday', 'Oklahoma City': 29, Sofia: 11, Bruges: 16 }, { Day: 'Wednesday', 'Oklahoma City': 27, Sofia: 8, Bruges: 29 }, { Day: 'Thursday', 'Oklahoma City': 37, Sofia: 14, Bruges: 29 }, { Day: 'Friday', 'Oklahoma City': 37, Sofia: 11, Bruges: 27 }, { Day: 'Saturday', 'Oklahoma City': 39, Sofia: 6, Bruges: 24 }, { Day: 'Sunday', 'Oklahoma City': 42, Sofia: 8, Bruges: 21 } ]; Smart('#chart', class { get properties() { return { borderLineColor: '#808080', backgroundImage: '../../../documentation/images/chart-custom-element-background.png', caption: 'Wind Speed', description: 'around the world', showLegend: true, padding: { left: 5, top: 5, right: 5, bottom: 5 }, titlePadding: { left: 90, top: 0, right: 0, bottom: 10 }, dataSource: sampleData, xAxis: { dataField: 'Day', gridLines: { visible: true, color: '#404040', step: 1 }, tickMarks: { visible: true, color: '#000000', step: 2 }, valuesOnTicks: false }, valueAxis: { description: 'Time in minutes', gridLines: { visible: true, color: '#404040', step: 2 }, tickMarks: { visible: true, color: '#000000', step: 1 }, axisSize: 'auto', tickMarksColor: '#888888' }, seriesGroups: [ { type: 'column', useGradientColors: true, series: [ { dataField: 'Sofia', fillColor: '#048BA8' }, { dataField: 'Bruges', fillColor: '#16DB93' } ] }, { type: 'stackedline', series: [ { dataField: 'Oklahoma City', symbolType: 'circle', symbolSize: 10, fillColorSymbol: '#3587CE', fillColor: '#3587CE', lineColor: '#0459A8' } ] } ] } } }); </script> </head> <body> <smart-chart id="chart"></smart-chart> </body> </html>